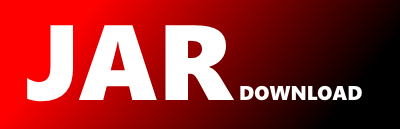
com.salesforce.servicelibs.ReactorChatGrpc Maven / Gradle / Ivy
package com.salesforce.servicelibs;
import static com.salesforce.servicelibs.ChatGrpc.getServiceDescriptor;
import static io.grpc.stub.ServerCalls.asyncUnaryCall;
import static io.grpc.stub.ServerCalls.asyncServerStreamingCall;
import static io.grpc.stub.ServerCalls.asyncClientStreamingCall;
import static io.grpc.stub.ServerCalls.asyncBidiStreamingCall;
@javax.annotation.Generated(
value = "by ReactorGrpc generator",
comments = "Source: Chat.proto")
public final class ReactorChatGrpc {
private ReactorChatGrpc() {}
public static ReactorChatStub newReactorStub(io.grpc.Channel channel) {
return new ReactorChatStub(channel);
}
public static final class ReactorChatStub {
private ChatGrpc.ChatStub delegateStub;
private ReactorChatStub(io.grpc.Channel channel) {
delegateStub = ChatGrpc.newStub(channel);
}
public reactor.core.publisher.Mono postMessage(reactor.core.publisher.Mono reactorRequest) {
return com.salesforce.reactorgrpc.stub.ClientCalls.oneToOne(reactorRequest, delegateStub::postMessage);
}
public reactor.core.publisher.Flux getMessages(reactor.core.publisher.Mono reactorRequest) {
return com.salesforce.reactorgrpc.stub.ClientCalls.oneToMany(reactorRequest, delegateStub::getMessages);
}
}
public static abstract class ChatImplBase implements io.grpc.BindableService {
public reactor.core.publisher.Mono postMessage(reactor.core.publisher.Mono request) {
throw new io.grpc.StatusRuntimeException(io.grpc.Status.UNIMPLEMENTED);
}
public reactor.core.publisher.Flux getMessages(reactor.core.publisher.Mono request) {
throw new io.grpc.StatusRuntimeException(io.grpc.Status.UNIMPLEMENTED);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
com.salesforce.servicelibs.ChatGrpc.METHOD_POST_MESSAGE,
asyncUnaryCall(
new MethodHandlers<
com.salesforce.servicelibs.ChatProto.ChatMessage,
com.google.protobuf.Empty>(
this, METHODID_POST_MESSAGE)))
.addMethod(
com.salesforce.servicelibs.ChatGrpc.METHOD_GET_MESSAGES,
asyncServerStreamingCall(
new MethodHandlers<
com.google.protobuf.Empty,
com.salesforce.servicelibs.ChatProto.ChatMessage>(
this, METHODID_GET_MESSAGES)))
.build();
}
}
private static final int METHODID_POST_MESSAGE = 0;
private static final int METHODID_GET_MESSAGES = 1;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final ChatImplBase serviceImpl;
private final int methodId;
MethodHandlers(ChatImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_POST_MESSAGE:
com.salesforce.reactorgrpc.stub.ServerCalls.oneToOne((com.salesforce.servicelibs.ChatProto.ChatMessage) request,
(io.grpc.stub.StreamObserver) responseObserver,
serviceImpl::postMessage);
break;
case METHODID_GET_MESSAGES:
com.salesforce.reactorgrpc.stub.ServerCalls.oneToMany((com.google.protobuf.Empty) request,
(io.grpc.stub.StreamObserver) responseObserver,
serviceImpl::getMessages);
break;
default:
throw new java.lang.AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new java.lang.AssertionError();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy