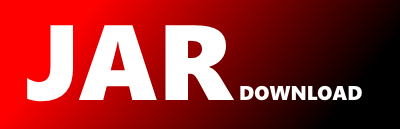
com.salesforce.reactorgrpc.GrpcRetry Maven / Gradle / Ivy
/*
* Copyright (c) 2019, Salesforce.com, Inc.
* All rights reserved.
* Licensed under the BSD 3-Clause license.
* For full license text, see LICENSE.txt file in the repo root or https://opensource.org/licenses/BSD-3-Clause
*/
package com.salesforce.reactorgrpc;
import org.reactivestreams.Publisher;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
import java.time.Duration;
import java.util.function.Function;
/**
* {@code GrpcRetry} is used to transparently re-establish a streaming gRPC request in the event of a server error.
*
* During a retry, the upstream rx pipeline is re-subscribed to acquire a request message and the RPC call re-issued.
* The downstream rx pipeline never sees the error.
*/
public final class GrpcRetry {
private GrpcRetry() { }
/**
* {@link GrpcRetry} functions for streaming response gRPC operations.
*/
public static final class OneToMany {
private OneToMany() {
}
/**
* Retries a streaming gRPC call, using the same semantics as {@link Flux#retryWhen(Function)}.
*
* For easier use, use the Retry builder from
* Reactor Extras.
*
* @param operation the gRPC operation to retry, typically from a generated reactive-grpc stub class
* @param whenFactory receives a Publisher of notifications with which a user can complete or error, aborting the retry
* @param I
* @param O
*
* @see Flux#retryWhen(Function)
*/
public static Function super Mono, Flux> retryWhen(final Function, Flux> operation, final Function, ? extends Publisher>> whenFactory) {
return request -> Flux.defer(() -> operation.apply(request)).retryWhen(whenFactory);
}
/**
* Retries a streaming gRPC call with a fixed delay between retries.
*
* @param operation the gRPC operation to retry, typically from a generated reactive-grpc stub class
* @param delay the delay between retries
* @param I
* @param O
*/
public static Function super Mono, Flux> retryAfter(final Function, Flux> operation, final Duration delay) {
return retryWhen(operation, errors -> errors.delayElements(delay));
}
/**
* Retries a streaming gRPC call immediately.
*
* @param operation the gRPC operation to retry, typically from a generated reactive-grpc stub class
* @param I
* @param O
*/
public static Function super Mono, Flux> retryImmediately(final Function, Flux> operation) {
return retryWhen(operation, errors -> errors);
}
}
/**
* {@link GrpcRetry} functions for bi-directional streaming gRPC operations.
*/
public static final class ManyToMany {
private ManyToMany() {
}
/**
* Retries a streaming gRPC call, using the same semantics as {@link Flux#retryWhen(Function)}.
*
* For easier use, use the Retry builder from
* Reactor Extras.
*
* @param operation the gRPC operation to retry, typically from a generated reactive-grpc stub class
* @param whenFactory receives a Publisher of notifications with which a user can complete or error, aborting the retry
* @param I
* @param O
*
* @see Flux#retryWhen(Function)
*/
public static Function super Flux, ? extends Publisher> retryWhen(final Function, Flux> operation, final Function, ? extends Publisher>> whenFactory) {
return request -> Flux.defer(() -> operation.apply(request)).retryWhen(whenFactory);
}
/**
* Retries a streaming gRPC call with a fixed delay between retries.
*
* @param operation the gRPC operation to retry, typically from a generated reactive-grpc stub class
* @param delay the delay between retries
* @param I
* @param O
*/
public static Function super Flux, ? extends Publisher> retryAfter(final Function, Flux> operation, final Duration delay) {
return retryWhen(operation, errors -> errors.delayElements(delay));
}
/**
* Retries a streaming gRPC call immediately.
*
* @param operation the gRPC operation to retry, typically from a generated reactive-grpc stub class
* @param I
* @param O
*/
public static Function super Flux, ? extends Publisher> retryImmediately(final Function, Flux> operation) {
return retryWhen(operation, errors -> errors);
}
}
/**
* {@link GrpcRetry} functions for streaming request gRPC operations.
*/
public static final class ManyToOne {
private ManyToOne() {
}
/**
* Retries a streaming gRPC call, using the same semantics as {@link Flux#retryWhen(Function)}.
*
* For easier use, use the Retry builder from
* Reactor Extras.
*
* @param operation the gRPC operation to retry, typically from a generated reactive-grpc stub class
* @param whenFactory receives a Publisher of notifications with which a user can complete or error, aborting the retry
* @param I
* @param O
*
* @see Flux#retryWhen(Function)
*/
public static Function super Flux, Mono> retryWhen(final Function, Mono> operation, final Function, ? extends Publisher>> whenFactory) {
return request -> Mono.defer(() -> operation.apply(request)).retryWhen(whenFactory);
}
/**
* Retries a streaming gRPC call with a fixed delay between retries.
*
* @param operation the gRPC operation to retry, typically from a generated reactive-grpc stub class
* @param delay the delay between retries
* @param I
* @param O
*/
public static Function super Flux, Mono> retryAfter(final Function, Mono> operation, final Duration delay) {
return retryWhen(operation, errors -> errors.delayElements(delay));
}
/**
* Retries a streaming gRPC call immediately.
*
* @param operation the gRPC operation to retry, typically from a generated reactive-grpc stub class
* @param I
* @param O
*/
public static Function super Flux, Mono> retryImmediately(final Function, Mono> operation) {
return retryWhen(operation, errors -> errors);
}
}
}