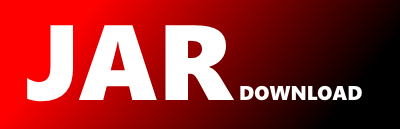
com.samskivert.depot.impl.MySQLBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of depot Show documentation
Show all versions of depot Show documentation
A library for relational-database-based persistence in Java.
//
// Depot library - a Java relational persistence library
// http://code.google.com/p/depot/source/browse/trunk/LICENSE
package com.samskivert.depot.impl;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.Map;
import java.util.Set;
import com.samskivert.depot.clause.Distinct;
import com.samskivert.util.StringUtil;
import com.samskivert.depot.PersistentRecord;
import com.samskivert.depot.annotation.FullTextIndex;
import com.samskivert.depot.clause.OrderBy;
import com.samskivert.depot.expression.ColumnExp;
import com.samskivert.depot.operator.FullText;
import com.samskivert.depot.impl.clause.DeleteClause;
import com.samskivert.depot.impl.clause.DropIndexClause;
import com.samskivert.depot.impl.expression.DateFun.DatePart;
import com.samskivert.depot.impl.expression.DateFun.DateTruncate;
import com.samskivert.depot.impl.expression.DateFun.DatePart.Part;
import com.samskivert.depot.impl.expression.NumericalFun.Trunc;
import static com.samskivert.Log.log;
public class MySQLBuilder
extends SQLBuilder
{
public class MSBuildVisitor extends BuildVisitor
{
@Override public Void visit (FullText.Match match)
{
renderMatch(match.getDefinition());
return null;
}
@Override public Void visit (FullText.Rank rank)
{
renderMatch(rank.getDefinition());
return null;
}
@Override public Void visit (DeleteClause deleteClause)
{
_builder.append("delete from ");
appendTableName(deleteClause.getPersistentClass());
_builder.append(" ");
// MySQL can't do DELETE FROM SomeTable AS T1, so we turn off abbreviations briefly.
boolean savedFlag = _types.getUseTableAbbreviations();
_types.setUseTableAbbreviations(false);
try {
deleteClause.getWhereClause().accept(this);
if (deleteClause.getLimit() != null) {
deleteClause.getLimit().accept(this);
}
} finally {
_types.setUseTableAbbreviations(savedFlag);
}
return null;
}
@Override public Void visit (Trunc> exp)
{
return appendFunctionCall("truncate", exp.getArg());
}
public Void visit (Distinct distinct)
{
if (distinct.getDistinctOn() != null) {
throw new IllegalArgumentException("MySQL does not support DISTINCT ON");
}
return super.visit(distinct);
}
@Override public Void visit (DatePart exp) {
return appendFunctionCall(getDateFunction(exp.getPart()), exp.getArg());
}
@Override
public Void visit (DateTruncate exp)
{
// exp.getTruncation() is currently always DAY
_builder.append(" cast(");
appendFunctionCall("date", exp.getArg());
_builder.append(" as datetime)");
return null;
}
protected String getDateFunction (Part part)
{
switch(part) {
case DAY_OF_MONTH:
return "dayofmonth";
case DAY_OF_WEEK:
return "dayofweek";
case DAY_OF_YEAR:
return "dayofyear";
case HOUR:
return "hour";
case MINUTE:
return "minute";
case MONTH:
return "month";
case SECOND:
return "second";
case WEEK:
return "week";
case YEAR:
return "year";
case EPOCH:
return "unix_timestamp";
}
throw new IllegalArgumentException("Unknown date part: " + part);
}
@Override
public Void visit (DropIndexClause dropIndexClause)
{
// MySQL's indexes are scoped on the table, not on the database, and the
// SQL syntax reflects it: DROP INDEX fooIx on fooTable
_builder.append("drop index ");
appendIdentifier(dropIndexClause.getName());
_builder.append(" on ");
appendTableName(dropIndexClause.getPersistentClass());
return null;
}
protected MSBuildVisitor (DepotTypes types)
{
super(types, false);
}
@Override protected void appendTableName (Class extends PersistentRecord> type)
{
_builder.append(_types.getTableName(type));
}
@Override protected void appendTableAbbreviation (Class extends PersistentRecord> type)
{
_builder.append(_types.getTableAbbreviation(type));
}
@Override protected void appendIdentifier (String field)
{
_builder.append("`").append(field).append("`");
}
protected void renderMatch (FullText fullText)
{
_builder.append("match(");
Class extends PersistentRecord> pClass = fullText.getPersistentClass();
String[] fields = _types.getMarshaller(pClass).getFullTextIndex(
fullText.getName()).fields();
for (int ii = 0; ii < fields.length; ii ++) {
if (ii > 0) {
_builder.append(", ");
}
new ColumnExp
© 2015 - 2025 Weber Informatics LLC | Privacy Policy