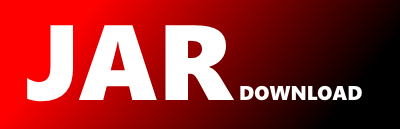
com.sandpolis.core.soi.Dependency Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sandpolis-core-soi Show documentation
Show all versions of sandpolis-core-soi Show documentation
The serial object injection library module
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: com/sandpolis/core/soi/Dependency.proto
package com.sandpolis.core.soi;
public final class Dependency {
private Dependency() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface SO_DependencyMatrixOrBuilder extends
// @@protoc_insertion_point(interface_extends:soi.SO_DependencyMatrix)
com.google.protobuf.MessageOrBuilder {
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
java.util.List
getArtifactList();
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact getArtifact(int index);
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
int getArtifactCount();
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
java.util.List extends com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.ArtifactOrBuilder>
getArtifactOrBuilderList();
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.ArtifactOrBuilder getArtifactOrBuilder(
int index);
}
/**
*
**
* The SO_DependencyMatrix object contains exhaustive dependency information about
* each instance.
*
*
* Protobuf type {@code soi.SO_DependencyMatrix}
*/
public static final class SO_DependencyMatrix extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:soi.SO_DependencyMatrix)
SO_DependencyMatrixOrBuilder {
private static final long serialVersionUID = 0L;
// Use SO_DependencyMatrix.newBuilder() to construct.
private SO_DependencyMatrix(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SO_DependencyMatrix() {
artifact_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SO_DependencyMatrix(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
artifact_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
artifact_.add(
input.readMessage(com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
artifact_ = java.util.Collections.unmodifiableList(artifact_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sandpolis.core.soi.Dependency.internal_static_soi_SO_DependencyMatrix_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sandpolis.core.soi.Dependency.internal_static_soi_SO_DependencyMatrix_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.class, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Builder.class);
}
public interface ArtifactOrBuilder extends
// @@protoc_insertion_point(interface_extends:soi.SO_DependencyMatrix.Artifact)
com.google.protobuf.MessageOrBuilder {
/**
*
* The artifact's repository coordinates if applicable.
*
*
* string coordinates = 1;
*/
java.lang.String getCoordinates();
/**
*
* The artifact's repository coordinates if applicable.
*
*
* string coordinates = 1;
*/
com.google.protobuf.ByteString
getCoordinatesBytes();
/**
*
* The size of the artifact in bytes.
*
*
* int64 size = 2;
*/
long getSize();
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
java.util.List
getNativeComponentList();
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent getNativeComponent(int index);
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
int getNativeComponentCount();
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
java.util.List extends com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponentOrBuilder>
getNativeComponentOrBuilderList();
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponentOrBuilder getNativeComponentOrBuilder(
int index);
/**
*
* A list of artifact IDs that this artifact directly depends on.
*
*
* repeated int32 dependency = 5;
*/
java.util.List getDependencyList();
/**
*
* A list of artifact IDs that this artifact directly depends on.
*
*
* repeated int32 dependency = 5;
*/
int getDependencyCount();
/**
*
* A list of artifact IDs that this artifact directly depends on.
*
*
* repeated int32 dependency = 5;
*/
int getDependency(int index);
}
/**
* Protobuf type {@code soi.SO_DependencyMatrix.Artifact}
*/
public static final class Artifact extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:soi.SO_DependencyMatrix.Artifact)
ArtifactOrBuilder {
private static final long serialVersionUID = 0L;
// Use Artifact.newBuilder() to construct.
private Artifact(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Artifact() {
coordinates_ = "";
nativeComponent_ = java.util.Collections.emptyList();
dependency_ = emptyIntList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Artifact(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
coordinates_ = s;
break;
}
case 16: {
size_ = input.readInt64();
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
nativeComponent_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
nativeComponent_.add(
input.readMessage(com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.parser(), extensionRegistry));
break;
}
case 40: {
if (!((mutable_bitField0_ & 0x00000008) != 0)) {
dependency_ = newIntList();
mutable_bitField0_ |= 0x00000008;
}
dependency_.addInt(input.readInt32());
break;
}
case 42: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000008) != 0) && input.getBytesUntilLimit() > 0) {
dependency_ = newIntList();
mutable_bitField0_ |= 0x00000008;
}
while (input.getBytesUntilLimit() > 0) {
dependency_.addInt(input.readInt32());
}
input.popLimit(limit);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) != 0)) {
nativeComponent_ = java.util.Collections.unmodifiableList(nativeComponent_);
}
if (((mutable_bitField0_ & 0x00000008) != 0)) {
dependency_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sandpolis.core.soi.Dependency.internal_static_soi_SO_DependencyMatrix_Artifact_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sandpolis.core.soi.Dependency.internal_static_soi_SO_DependencyMatrix_Artifact_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.class, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.Builder.class);
}
public interface NativeComponentOrBuilder extends
// @@protoc_insertion_point(interface_extends:soi.SO_DependencyMatrix.Artifact.NativeComponent)
com.google.protobuf.MessageOrBuilder {
/**
*
* The component's compatible platform.
*util.OsType platform = 1;
*
*
* string platform = 1;
*/
java.lang.String getPlatform();
/**
*
* The component's compatible platform.
*util.OsType platform = 1;
*
*
* string platform = 1;
*/
com.google.protobuf.ByteString
getPlatformBytes();
/**
*
* The component's compatible system architecture.
*util.Architecture architecture = 2;
*
*
* string architecture = 2;
*/
java.lang.String getArchitecture();
/**
*
* The component's compatible system architecture.
*util.Architecture architecture = 2;
*
*
* string architecture = 2;
*/
com.google.protobuf.ByteString
getArchitectureBytes();
/**
*
* The component's internal path.
*
*
* string path = 3;
*/
java.lang.String getPath();
/**
*
* The component's internal path.
*
*
* string path = 3;
*/
com.google.protobuf.ByteString
getPathBytes();
/**
*
* The component's size in bytes
*
*
* int64 size = 4;
*/
long getSize();
}
/**
*
**
* An internal component of the artifact which is specific to a platform
* and system architecture.
*
*
* Protobuf type {@code soi.SO_DependencyMatrix.Artifact.NativeComponent}
*/
public static final class NativeComponent extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:soi.SO_DependencyMatrix.Artifact.NativeComponent)
NativeComponentOrBuilder {
private static final long serialVersionUID = 0L;
// Use NativeComponent.newBuilder() to construct.
private NativeComponent(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NativeComponent() {
platform_ = "";
architecture_ = "";
path_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NativeComponent(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
platform_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
architecture_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
path_ = s;
break;
}
case 32: {
size_ = input.readInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sandpolis.core.soi.Dependency.internal_static_soi_SO_DependencyMatrix_Artifact_NativeComponent_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sandpolis.core.soi.Dependency.internal_static_soi_SO_DependencyMatrix_Artifact_NativeComponent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.class, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.Builder.class);
}
public static final int PLATFORM_FIELD_NUMBER = 1;
private volatile java.lang.Object platform_;
/**
*
* The component's compatible platform.
*util.OsType platform = 1;
*
*
* string platform = 1;
*/
public java.lang.String getPlatform() {
java.lang.Object ref = platform_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
platform_ = s;
return s;
}
}
/**
*
* The component's compatible platform.
*util.OsType platform = 1;
*
*
* string platform = 1;
*/
public com.google.protobuf.ByteString
getPlatformBytes() {
java.lang.Object ref = platform_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
platform_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ARCHITECTURE_FIELD_NUMBER = 2;
private volatile java.lang.Object architecture_;
/**
*
* The component's compatible system architecture.
*util.Architecture architecture = 2;
*
*
* string architecture = 2;
*/
public java.lang.String getArchitecture() {
java.lang.Object ref = architecture_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
architecture_ = s;
return s;
}
}
/**
*
* The component's compatible system architecture.
*util.Architecture architecture = 2;
*
*
* string architecture = 2;
*/
public com.google.protobuf.ByteString
getArchitectureBytes() {
java.lang.Object ref = architecture_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
architecture_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PATH_FIELD_NUMBER = 3;
private volatile java.lang.Object path_;
/**
*
* The component's internal path.
*
*
* string path = 3;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
}
}
/**
*
* The component's internal path.
*
*
* string path = 3;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SIZE_FIELD_NUMBER = 4;
private long size_;
/**
*
* The component's size in bytes
*
*
* int64 size = 4;
*/
public long getSize() {
return size_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPlatformBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, platform_);
}
if (!getArchitectureBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, architecture_);
}
if (!getPathBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, path_);
}
if (size_ != 0L) {
output.writeInt64(4, size_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPlatformBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, platform_);
}
if (!getArchitectureBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, architecture_);
}
if (!getPathBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, path_);
}
if (size_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, size_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent)) {
return super.equals(obj);
}
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent other = (com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent) obj;
if (!getPlatform()
.equals(other.getPlatform())) return false;
if (!getArchitecture()
.equals(other.getArchitecture())) return false;
if (!getPath()
.equals(other.getPath())) return false;
if (getSize()
!= other.getSize()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PLATFORM_FIELD_NUMBER;
hash = (53 * hash) + getPlatform().hashCode();
hash = (37 * hash) + ARCHITECTURE_FIELD_NUMBER;
hash = (53 * hash) + getArchitecture().hashCode();
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
hash = (37 * hash) + SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSize());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* An internal component of the artifact which is specific to a platform
* and system architecture.
*
*
* Protobuf type {@code soi.SO_DependencyMatrix.Artifact.NativeComponent}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:soi.SO_DependencyMatrix.Artifact.NativeComponent)
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sandpolis.core.soi.Dependency.internal_static_soi_SO_DependencyMatrix_Artifact_NativeComponent_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sandpolis.core.soi.Dependency.internal_static_soi_SO_DependencyMatrix_Artifact_NativeComponent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.class, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.Builder.class);
}
// Construct using com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
platform_ = "";
architecture_ = "";
path_ = "";
size_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.sandpolis.core.soi.Dependency.internal_static_soi_SO_DependencyMatrix_Artifact_NativeComponent_descriptor;
}
@java.lang.Override
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent getDefaultInstanceForType() {
return com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.getDefaultInstance();
}
@java.lang.Override
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent build() {
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent buildPartial() {
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent result = new com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent(this);
result.platform_ = platform_;
result.architecture_ = architecture_;
result.path_ = path_;
result.size_ = size_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent) {
return mergeFrom((com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent other) {
if (other == com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.getDefaultInstance()) return this;
if (!other.getPlatform().isEmpty()) {
platform_ = other.platform_;
onChanged();
}
if (!other.getArchitecture().isEmpty()) {
architecture_ = other.architecture_;
onChanged();
}
if (!other.getPath().isEmpty()) {
path_ = other.path_;
onChanged();
}
if (other.getSize() != 0L) {
setSize(other.getSize());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object platform_ = "";
/**
*
* The component's compatible platform.
*util.OsType platform = 1;
*
*
* string platform = 1;
*/
public java.lang.String getPlatform() {
java.lang.Object ref = platform_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
platform_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The component's compatible platform.
*util.OsType platform = 1;
*
*
* string platform = 1;
*/
public com.google.protobuf.ByteString
getPlatformBytes() {
java.lang.Object ref = platform_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
platform_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The component's compatible platform.
*util.OsType platform = 1;
*
*
* string platform = 1;
*/
public Builder setPlatform(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
platform_ = value;
onChanged();
return this;
}
/**
*
* The component's compatible platform.
*util.OsType platform = 1;
*
*
* string platform = 1;
*/
public Builder clearPlatform() {
platform_ = getDefaultInstance().getPlatform();
onChanged();
return this;
}
/**
*
* The component's compatible platform.
*util.OsType platform = 1;
*
*
* string platform = 1;
*/
public Builder setPlatformBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
platform_ = value;
onChanged();
return this;
}
private java.lang.Object architecture_ = "";
/**
*
* The component's compatible system architecture.
*util.Architecture architecture = 2;
*
*
* string architecture = 2;
*/
public java.lang.String getArchitecture() {
java.lang.Object ref = architecture_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
architecture_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The component's compatible system architecture.
*util.Architecture architecture = 2;
*
*
* string architecture = 2;
*/
public com.google.protobuf.ByteString
getArchitectureBytes() {
java.lang.Object ref = architecture_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
architecture_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The component's compatible system architecture.
*util.Architecture architecture = 2;
*
*
* string architecture = 2;
*/
public Builder setArchitecture(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
architecture_ = value;
onChanged();
return this;
}
/**
*
* The component's compatible system architecture.
*util.Architecture architecture = 2;
*
*
* string architecture = 2;
*/
public Builder clearArchitecture() {
architecture_ = getDefaultInstance().getArchitecture();
onChanged();
return this;
}
/**
*
* The component's compatible system architecture.
*util.Architecture architecture = 2;
*
*
* string architecture = 2;
*/
public Builder setArchitectureBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
architecture_ = value;
onChanged();
return this;
}
private java.lang.Object path_ = "";
/**
*
* The component's internal path.
*
*
* string path = 3;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The component's internal path.
*
*
* string path = 3;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The component's internal path.
*
*
* string path = 3;
*/
public Builder setPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
path_ = value;
onChanged();
return this;
}
/**
*
* The component's internal path.
*
*
* string path = 3;
*/
public Builder clearPath() {
path_ = getDefaultInstance().getPath();
onChanged();
return this;
}
/**
*
* The component's internal path.
*
*
* string path = 3;
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
path_ = value;
onChanged();
return this;
}
private long size_ ;
/**
*
* The component's size in bytes
*
*
* int64 size = 4;
*/
public long getSize() {
return size_;
}
/**
*
* The component's size in bytes
*
*
* int64 size = 4;
*/
public Builder setSize(long value) {
size_ = value;
onChanged();
return this;
}
/**
*
* The component's size in bytes
*
*
* int64 size = 4;
*/
public Builder clearSize() {
size_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:soi.SO_DependencyMatrix.Artifact.NativeComponent)
}
// @@protoc_insertion_point(class_scope:soi.SO_DependencyMatrix.Artifact.NativeComponent)
private static final com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent();
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NativeComponent parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NativeComponent(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int COORDINATES_FIELD_NUMBER = 1;
private volatile java.lang.Object coordinates_;
/**
*
* The artifact's repository coordinates if applicable.
*
*
* string coordinates = 1;
*/
public java.lang.String getCoordinates() {
java.lang.Object ref = coordinates_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
coordinates_ = s;
return s;
}
}
/**
*
* The artifact's repository coordinates if applicable.
*
*
* string coordinates = 1;
*/
public com.google.protobuf.ByteString
getCoordinatesBytes() {
java.lang.Object ref = coordinates_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
coordinates_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SIZE_FIELD_NUMBER = 2;
private long size_;
/**
*
* The size of the artifact in bytes.
*
*
* int64 size = 2;
*/
public long getSize() {
return size_;
}
public static final int NATIVE_COMPONENT_FIELD_NUMBER = 4;
private java.util.List nativeComponent_;
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public java.util.List getNativeComponentList() {
return nativeComponent_;
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public java.util.List extends com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponentOrBuilder>
getNativeComponentOrBuilderList() {
return nativeComponent_;
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public int getNativeComponentCount() {
return nativeComponent_.size();
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent getNativeComponent(int index) {
return nativeComponent_.get(index);
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponentOrBuilder getNativeComponentOrBuilder(
int index) {
return nativeComponent_.get(index);
}
public static final int DEPENDENCY_FIELD_NUMBER = 5;
private com.google.protobuf.Internal.IntList dependency_;
/**
*
* A list of artifact IDs that this artifact directly depends on.
*
*
* repeated int32 dependency = 5;
*/
public java.util.List
getDependencyList() {
return dependency_;
}
/**
*
* A list of artifact IDs that this artifact directly depends on.
*
*
* repeated int32 dependency = 5;
*/
public int getDependencyCount() {
return dependency_.size();
}
/**
*
* A list of artifact IDs that this artifact directly depends on.
*
*
* repeated int32 dependency = 5;
*/
public int getDependency(int index) {
return dependency_.getInt(index);
}
private int dependencyMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (!getCoordinatesBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, coordinates_);
}
if (size_ != 0L) {
output.writeInt64(2, size_);
}
for (int i = 0; i < nativeComponent_.size(); i++) {
output.writeMessage(4, nativeComponent_.get(i));
}
if (getDependencyList().size() > 0) {
output.writeUInt32NoTag(42);
output.writeUInt32NoTag(dependencyMemoizedSerializedSize);
}
for (int i = 0; i < dependency_.size(); i++) {
output.writeInt32NoTag(dependency_.getInt(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getCoordinatesBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, coordinates_);
}
if (size_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, size_);
}
for (int i = 0; i < nativeComponent_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, nativeComponent_.get(i));
}
{
int dataSize = 0;
for (int i = 0; i < dependency_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dependency_.getInt(i));
}
size += dataSize;
if (!getDependencyList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
dependencyMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact)) {
return super.equals(obj);
}
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact other = (com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact) obj;
if (!getCoordinates()
.equals(other.getCoordinates())) return false;
if (getSize()
!= other.getSize()) return false;
if (!getNativeComponentList()
.equals(other.getNativeComponentList())) return false;
if (!getDependencyList()
.equals(other.getDependencyList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + COORDINATES_FIELD_NUMBER;
hash = (53 * hash) + getCoordinates().hashCode();
hash = (37 * hash) + SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSize());
if (getNativeComponentCount() > 0) {
hash = (37 * hash) + NATIVE_COMPONENT_FIELD_NUMBER;
hash = (53 * hash) + getNativeComponentList().hashCode();
}
if (getDependencyCount() > 0) {
hash = (37 * hash) + DEPENDENCY_FIELD_NUMBER;
hash = (53 * hash) + getDependencyList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code soi.SO_DependencyMatrix.Artifact}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:soi.SO_DependencyMatrix.Artifact)
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.ArtifactOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sandpolis.core.soi.Dependency.internal_static_soi_SO_DependencyMatrix_Artifact_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sandpolis.core.soi.Dependency.internal_static_soi_SO_DependencyMatrix_Artifact_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.class, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.Builder.class);
}
// Construct using com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getNativeComponentFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
coordinates_ = "";
size_ = 0L;
if (nativeComponentBuilder_ == null) {
nativeComponent_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
nativeComponentBuilder_.clear();
}
dependency_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.sandpolis.core.soi.Dependency.internal_static_soi_SO_DependencyMatrix_Artifact_descriptor;
}
@java.lang.Override
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact getDefaultInstanceForType() {
return com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.getDefaultInstance();
}
@java.lang.Override
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact build() {
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact buildPartial() {
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact result = new com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.coordinates_ = coordinates_;
result.size_ = size_;
if (nativeComponentBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
nativeComponent_ = java.util.Collections.unmodifiableList(nativeComponent_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.nativeComponent_ = nativeComponent_;
} else {
result.nativeComponent_ = nativeComponentBuilder_.build();
}
if (((bitField0_ & 0x00000008) != 0)) {
dependency_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000008);
}
result.dependency_ = dependency_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact) {
return mergeFrom((com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact other) {
if (other == com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.getDefaultInstance()) return this;
if (!other.getCoordinates().isEmpty()) {
coordinates_ = other.coordinates_;
onChanged();
}
if (other.getSize() != 0L) {
setSize(other.getSize());
}
if (nativeComponentBuilder_ == null) {
if (!other.nativeComponent_.isEmpty()) {
if (nativeComponent_.isEmpty()) {
nativeComponent_ = other.nativeComponent_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureNativeComponentIsMutable();
nativeComponent_.addAll(other.nativeComponent_);
}
onChanged();
}
} else {
if (!other.nativeComponent_.isEmpty()) {
if (nativeComponentBuilder_.isEmpty()) {
nativeComponentBuilder_.dispose();
nativeComponentBuilder_ = null;
nativeComponent_ = other.nativeComponent_;
bitField0_ = (bitField0_ & ~0x00000004);
nativeComponentBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getNativeComponentFieldBuilder() : null;
} else {
nativeComponentBuilder_.addAllMessages(other.nativeComponent_);
}
}
}
if (!other.dependency_.isEmpty()) {
if (dependency_.isEmpty()) {
dependency_ = other.dependency_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureDependencyIsMutable();
dependency_.addAll(other.dependency_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object coordinates_ = "";
/**
*
* The artifact's repository coordinates if applicable.
*
*
* string coordinates = 1;
*/
public java.lang.String getCoordinates() {
java.lang.Object ref = coordinates_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
coordinates_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The artifact's repository coordinates if applicable.
*
*
* string coordinates = 1;
*/
public com.google.protobuf.ByteString
getCoordinatesBytes() {
java.lang.Object ref = coordinates_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
coordinates_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The artifact's repository coordinates if applicable.
*
*
* string coordinates = 1;
*/
public Builder setCoordinates(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
coordinates_ = value;
onChanged();
return this;
}
/**
*
* The artifact's repository coordinates if applicable.
*
*
* string coordinates = 1;
*/
public Builder clearCoordinates() {
coordinates_ = getDefaultInstance().getCoordinates();
onChanged();
return this;
}
/**
*
* The artifact's repository coordinates if applicable.
*
*
* string coordinates = 1;
*/
public Builder setCoordinatesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
coordinates_ = value;
onChanged();
return this;
}
private long size_ ;
/**
*
* The size of the artifact in bytes.
*
*
* int64 size = 2;
*/
public long getSize() {
return size_;
}
/**
*
* The size of the artifact in bytes.
*
*
* int64 size = 2;
*/
public Builder setSize(long value) {
size_ = value;
onChanged();
return this;
}
/**
*
* The size of the artifact in bytes.
*
*
* int64 size = 2;
*/
public Builder clearSize() {
size_ = 0L;
onChanged();
return this;
}
private java.util.List nativeComponent_ =
java.util.Collections.emptyList();
private void ensureNativeComponentIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
nativeComponent_ = new java.util.ArrayList(nativeComponent_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.Builder, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponentOrBuilder> nativeComponentBuilder_;
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public java.util.List getNativeComponentList() {
if (nativeComponentBuilder_ == null) {
return java.util.Collections.unmodifiableList(nativeComponent_);
} else {
return nativeComponentBuilder_.getMessageList();
}
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public int getNativeComponentCount() {
if (nativeComponentBuilder_ == null) {
return nativeComponent_.size();
} else {
return nativeComponentBuilder_.getCount();
}
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent getNativeComponent(int index) {
if (nativeComponentBuilder_ == null) {
return nativeComponent_.get(index);
} else {
return nativeComponentBuilder_.getMessage(index);
}
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public Builder setNativeComponent(
int index, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent value) {
if (nativeComponentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNativeComponentIsMutable();
nativeComponent_.set(index, value);
onChanged();
} else {
nativeComponentBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public Builder setNativeComponent(
int index, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.Builder builderForValue) {
if (nativeComponentBuilder_ == null) {
ensureNativeComponentIsMutable();
nativeComponent_.set(index, builderForValue.build());
onChanged();
} else {
nativeComponentBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public Builder addNativeComponent(com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent value) {
if (nativeComponentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNativeComponentIsMutable();
nativeComponent_.add(value);
onChanged();
} else {
nativeComponentBuilder_.addMessage(value);
}
return this;
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public Builder addNativeComponent(
int index, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent value) {
if (nativeComponentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNativeComponentIsMutable();
nativeComponent_.add(index, value);
onChanged();
} else {
nativeComponentBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public Builder addNativeComponent(
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.Builder builderForValue) {
if (nativeComponentBuilder_ == null) {
ensureNativeComponentIsMutable();
nativeComponent_.add(builderForValue.build());
onChanged();
} else {
nativeComponentBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public Builder addNativeComponent(
int index, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.Builder builderForValue) {
if (nativeComponentBuilder_ == null) {
ensureNativeComponentIsMutable();
nativeComponent_.add(index, builderForValue.build());
onChanged();
} else {
nativeComponentBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public Builder addAllNativeComponent(
java.lang.Iterable extends com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent> values) {
if (nativeComponentBuilder_ == null) {
ensureNativeComponentIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, nativeComponent_);
onChanged();
} else {
nativeComponentBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public Builder clearNativeComponent() {
if (nativeComponentBuilder_ == null) {
nativeComponent_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
nativeComponentBuilder_.clear();
}
return this;
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public Builder removeNativeComponent(int index) {
if (nativeComponentBuilder_ == null) {
ensureNativeComponentIsMutable();
nativeComponent_.remove(index);
onChanged();
} else {
nativeComponentBuilder_.remove(index);
}
return this;
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.Builder getNativeComponentBuilder(
int index) {
return getNativeComponentFieldBuilder().getBuilder(index);
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponentOrBuilder getNativeComponentOrBuilder(
int index) {
if (nativeComponentBuilder_ == null) {
return nativeComponent_.get(index); } else {
return nativeComponentBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public java.util.List extends com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponentOrBuilder>
getNativeComponentOrBuilderList() {
if (nativeComponentBuilder_ != null) {
return nativeComponentBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(nativeComponent_);
}
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.Builder addNativeComponentBuilder() {
return getNativeComponentFieldBuilder().addBuilder(
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.getDefaultInstance());
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.Builder addNativeComponentBuilder(
int index) {
return getNativeComponentFieldBuilder().addBuilder(
index, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.getDefaultInstance());
}
/**
*
* The artifact's internal native components.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact.NativeComponent native_component = 4;
*/
public java.util.List
getNativeComponentBuilderList() {
return getNativeComponentFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.Builder, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponentOrBuilder>
getNativeComponentFieldBuilder() {
if (nativeComponentBuilder_ == null) {
nativeComponentBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponent.Builder, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.NativeComponentOrBuilder>(
nativeComponent_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
nativeComponent_ = null;
}
return nativeComponentBuilder_;
}
private com.google.protobuf.Internal.IntList dependency_ = emptyIntList();
private void ensureDependencyIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
dependency_ = mutableCopy(dependency_);
bitField0_ |= 0x00000008;
}
}
/**
*
* A list of artifact IDs that this artifact directly depends on.
*
*
* repeated int32 dependency = 5;
*/
public java.util.List
getDependencyList() {
return ((bitField0_ & 0x00000008) != 0) ?
java.util.Collections.unmodifiableList(dependency_) : dependency_;
}
/**
*
* A list of artifact IDs that this artifact directly depends on.
*
*
* repeated int32 dependency = 5;
*/
public int getDependencyCount() {
return dependency_.size();
}
/**
*
* A list of artifact IDs that this artifact directly depends on.
*
*
* repeated int32 dependency = 5;
*/
public int getDependency(int index) {
return dependency_.getInt(index);
}
/**
*
* A list of artifact IDs that this artifact directly depends on.
*
*
* repeated int32 dependency = 5;
*/
public Builder setDependency(
int index, int value) {
ensureDependencyIsMutable();
dependency_.setInt(index, value);
onChanged();
return this;
}
/**
*
* A list of artifact IDs that this artifact directly depends on.
*
*
* repeated int32 dependency = 5;
*/
public Builder addDependency(int value) {
ensureDependencyIsMutable();
dependency_.addInt(value);
onChanged();
return this;
}
/**
*
* A list of artifact IDs that this artifact directly depends on.
*
*
* repeated int32 dependency = 5;
*/
public Builder addAllDependency(
java.lang.Iterable extends java.lang.Integer> values) {
ensureDependencyIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, dependency_);
onChanged();
return this;
}
/**
*
* A list of artifact IDs that this artifact directly depends on.
*
*
* repeated int32 dependency = 5;
*/
public Builder clearDependency() {
dependency_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:soi.SO_DependencyMatrix.Artifact)
}
// @@protoc_insertion_point(class_scope:soi.SO_DependencyMatrix.Artifact)
private static final com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact();
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Artifact parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Artifact(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int ARTIFACT_FIELD_NUMBER = 1;
private java.util.List artifact_;
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public java.util.List getArtifactList() {
return artifact_;
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public java.util.List extends com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.ArtifactOrBuilder>
getArtifactOrBuilderList() {
return artifact_;
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public int getArtifactCount() {
return artifact_.size();
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact getArtifact(int index) {
return artifact_.get(index);
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.ArtifactOrBuilder getArtifactOrBuilder(
int index) {
return artifact_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < artifact_.size(); i++) {
output.writeMessage(1, artifact_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < artifact_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, artifact_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.sandpolis.core.soi.Dependency.SO_DependencyMatrix)) {
return super.equals(obj);
}
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix other = (com.sandpolis.core.soi.Dependency.SO_DependencyMatrix) obj;
if (!getArtifactList()
.equals(other.getArtifactList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getArtifactCount() > 0) {
hash = (37 * hash) + ARTIFACT_FIELD_NUMBER;
hash = (53 * hash) + getArtifactList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.sandpolis.core.soi.Dependency.SO_DependencyMatrix prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* The SO_DependencyMatrix object contains exhaustive dependency information about
* each instance.
*
*
* Protobuf type {@code soi.SO_DependencyMatrix}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:soi.SO_DependencyMatrix)
com.sandpolis.core.soi.Dependency.SO_DependencyMatrixOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.sandpolis.core.soi.Dependency.internal_static_soi_SO_DependencyMatrix_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.sandpolis.core.soi.Dependency.internal_static_soi_SO_DependencyMatrix_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.class, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Builder.class);
}
// Construct using com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getArtifactFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (artifactBuilder_ == null) {
artifact_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
artifactBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.sandpolis.core.soi.Dependency.internal_static_soi_SO_DependencyMatrix_descriptor;
}
@java.lang.Override
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix getDefaultInstanceForType() {
return com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.getDefaultInstance();
}
@java.lang.Override
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix build() {
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix buildPartial() {
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix result = new com.sandpolis.core.soi.Dependency.SO_DependencyMatrix(this);
int from_bitField0_ = bitField0_;
if (artifactBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
artifact_ = java.util.Collections.unmodifiableList(artifact_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.artifact_ = artifact_;
} else {
result.artifact_ = artifactBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.sandpolis.core.soi.Dependency.SO_DependencyMatrix) {
return mergeFrom((com.sandpolis.core.soi.Dependency.SO_DependencyMatrix)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.sandpolis.core.soi.Dependency.SO_DependencyMatrix other) {
if (other == com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.getDefaultInstance()) return this;
if (artifactBuilder_ == null) {
if (!other.artifact_.isEmpty()) {
if (artifact_.isEmpty()) {
artifact_ = other.artifact_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureArtifactIsMutable();
artifact_.addAll(other.artifact_);
}
onChanged();
}
} else {
if (!other.artifact_.isEmpty()) {
if (artifactBuilder_.isEmpty()) {
artifactBuilder_.dispose();
artifactBuilder_ = null;
artifact_ = other.artifact_;
bitField0_ = (bitField0_ & ~0x00000001);
artifactBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getArtifactFieldBuilder() : null;
} else {
artifactBuilder_.addAllMessages(other.artifact_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.sandpolis.core.soi.Dependency.SO_DependencyMatrix) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List artifact_ =
java.util.Collections.emptyList();
private void ensureArtifactIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
artifact_ = new java.util.ArrayList(artifact_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.Builder, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.ArtifactOrBuilder> artifactBuilder_;
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public java.util.List getArtifactList() {
if (artifactBuilder_ == null) {
return java.util.Collections.unmodifiableList(artifact_);
} else {
return artifactBuilder_.getMessageList();
}
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public int getArtifactCount() {
if (artifactBuilder_ == null) {
return artifact_.size();
} else {
return artifactBuilder_.getCount();
}
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact getArtifact(int index) {
if (artifactBuilder_ == null) {
return artifact_.get(index);
} else {
return artifactBuilder_.getMessage(index);
}
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public Builder setArtifact(
int index, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact value) {
if (artifactBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArtifactIsMutable();
artifact_.set(index, value);
onChanged();
} else {
artifactBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public Builder setArtifact(
int index, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.Builder builderForValue) {
if (artifactBuilder_ == null) {
ensureArtifactIsMutable();
artifact_.set(index, builderForValue.build());
onChanged();
} else {
artifactBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public Builder addArtifact(com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact value) {
if (artifactBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArtifactIsMutable();
artifact_.add(value);
onChanged();
} else {
artifactBuilder_.addMessage(value);
}
return this;
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public Builder addArtifact(
int index, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact value) {
if (artifactBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArtifactIsMutable();
artifact_.add(index, value);
onChanged();
} else {
artifactBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public Builder addArtifact(
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.Builder builderForValue) {
if (artifactBuilder_ == null) {
ensureArtifactIsMutable();
artifact_.add(builderForValue.build());
onChanged();
} else {
artifactBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public Builder addArtifact(
int index, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.Builder builderForValue) {
if (artifactBuilder_ == null) {
ensureArtifactIsMutable();
artifact_.add(index, builderForValue.build());
onChanged();
} else {
artifactBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public Builder addAllArtifact(
java.lang.Iterable extends com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact> values) {
if (artifactBuilder_ == null) {
ensureArtifactIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, artifact_);
onChanged();
} else {
artifactBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public Builder clearArtifact() {
if (artifactBuilder_ == null) {
artifact_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
artifactBuilder_.clear();
}
return this;
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public Builder removeArtifact(int index) {
if (artifactBuilder_ == null) {
ensureArtifactIsMutable();
artifact_.remove(index);
onChanged();
} else {
artifactBuilder_.remove(index);
}
return this;
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.Builder getArtifactBuilder(
int index) {
return getArtifactFieldBuilder().getBuilder(index);
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.ArtifactOrBuilder getArtifactOrBuilder(
int index) {
if (artifactBuilder_ == null) {
return artifact_.get(index); } else {
return artifactBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public java.util.List extends com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.ArtifactOrBuilder>
getArtifactOrBuilderList() {
if (artifactBuilder_ != null) {
return artifactBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(artifact_);
}
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.Builder addArtifactBuilder() {
return getArtifactFieldBuilder().addBuilder(
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.getDefaultInstance());
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.Builder addArtifactBuilder(
int index) {
return getArtifactFieldBuilder().addBuilder(
index, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.getDefaultInstance());
}
/**
*
* A list of every artifact where the artifact ID is its position in the list.
*
*
* repeated .soi.SO_DependencyMatrix.Artifact artifact = 1;
*/
public java.util.List
getArtifactBuilderList() {
return getArtifactFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.Builder, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.ArtifactOrBuilder>
getArtifactFieldBuilder() {
if (artifactBuilder_ == null) {
artifactBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.Artifact.Builder, com.sandpolis.core.soi.Dependency.SO_DependencyMatrix.ArtifactOrBuilder>(
artifact_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
artifact_ = null;
}
return artifactBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:soi.SO_DependencyMatrix)
}
// @@protoc_insertion_point(class_scope:soi.SO_DependencyMatrix)
private static final com.sandpolis.core.soi.Dependency.SO_DependencyMatrix DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.sandpolis.core.soi.Dependency.SO_DependencyMatrix();
}
public static com.sandpolis.core.soi.Dependency.SO_DependencyMatrix getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SO_DependencyMatrix parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SO_DependencyMatrix(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.sandpolis.core.soi.Dependency.SO_DependencyMatrix getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_soi_SO_DependencyMatrix_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_soi_SO_DependencyMatrix_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_soi_SO_DependencyMatrix_Artifact_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_soi_SO_DependencyMatrix_Artifact_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_soi_SO_DependencyMatrix_Artifact_NativeComponent_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_soi_SO_DependencyMatrix_Artifact_NativeComponent_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\'com/sandpolis/core/soi/Dependency.prot" +
"o\022\003soi\"\262\002\n\023SO_DependencyMatrix\0223\n\010artifa" +
"ct\030\001 \003(\0132!.soi.SO_DependencyMatrix.Artif" +
"act\032\345\001\n\010Artifact\022\023\n\013coordinates\030\001 \001(\t\022\014\n" +
"\004size\030\002 \001(\003\022K\n\020native_component\030\004 \003(\01321." +
"soi.SO_DependencyMatrix.Artifact.NativeC" +
"omponent\022\022\n\ndependency\030\005 \003(\005\032U\n\017NativeCo" +
"mponent\022\020\n\010platform\030\001 \001(\t\022\024\n\014architectur" +
"e\030\002 \001(\t\022\014\n\004path\030\003 \001(\t\022\014\n\004size\030\004 \001(\003B\030\n\026c" +
"om.sandpolis.core.soib\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
internal_static_soi_SO_DependencyMatrix_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_soi_SO_DependencyMatrix_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_soi_SO_DependencyMatrix_descriptor,
new java.lang.String[] { "Artifact", });
internal_static_soi_SO_DependencyMatrix_Artifact_descriptor =
internal_static_soi_SO_DependencyMatrix_descriptor.getNestedTypes().get(0);
internal_static_soi_SO_DependencyMatrix_Artifact_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_soi_SO_DependencyMatrix_Artifact_descriptor,
new java.lang.String[] { "Coordinates", "Size", "NativeComponent", "Dependency", });
internal_static_soi_SO_DependencyMatrix_Artifact_NativeComponent_descriptor =
internal_static_soi_SO_DependencyMatrix_Artifact_descriptor.getNestedTypes().get(0);
internal_static_soi_SO_DependencyMatrix_Artifact_NativeComponent_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_soi_SO_DependencyMatrix_Artifact_NativeComponent_descriptor,
new java.lang.String[] { "Platform", "Architecture", "Path", "Size", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy