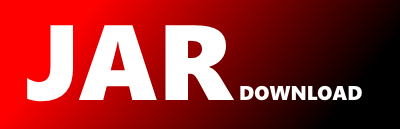
com.sandpolis.server.vanilla.store.group.GroupStore Maven / Gradle / Ivy
/******************************************************************************
* *
* Copyright 2018 Subterranean Security *
* *
* Licensed under the Apache License, Version 2.0 (the "License"); *
* you may not use this file except in compliance with the License. *
* You may obtain a copy of the License at *
* *
* http://www.apache.org/licenses/LICENSE-2.0 *
* *
* Unless required by applicable law or agreed to in writing, software *
* distributed under the License is distributed on an "AS IS" BASIS, *
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. *
* See the License for the specific language governing permissions and *
* limitations under the License. *
* *
*****************************************************************************/
package com.sandpolis.server.vanilla.store.group;
import static com.google.common.base.Preconditions.checkArgument;
import static com.sandpolis.core.util.ProtoUtil.begin;
import static com.sandpolis.core.util.ProtoUtil.failure;
import static com.sandpolis.core.util.ProtoUtil.success;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.sandpolis.core.instance.Store;
import com.sandpolis.core.instance.Store.ManualInitializer;
import com.sandpolis.core.instance.storage.StoreProvider;
import com.sandpolis.core.instance.storage.StoreProviderFactory;
import com.sandpolis.core.instance.storage.database.Database;
import com.sandpolis.core.proto.pojo.Group.GroupConfig;
import com.sandpolis.core.proto.pojo.Group.ProtoGroup;
import com.sandpolis.core.proto.util.Result.ErrorCode;
import com.sandpolis.core.proto.util.Result.Outcome;
import com.sandpolis.core.util.ValidationUtil;
import com.sandpolis.server.vanilla.store.user.User;
/**
* The {@link GroupStore} manages groups and authentication mechanisms.
*
* @author cilki
* @since 5.0.0
*/
@ManualInitializer
public final class GroupStore extends Store {
private static final Logger log = LoggerFactory.getLogger(GroupStore.class);
private static StoreProvider provider;
public static void init(StoreProvider provider) {
GroupStore.provider = Objects.requireNonNull(provider);
if (log.isDebugEnabled())
log.debug("Initialized store containing {} entities", provider.count());
}
public static void load(Database main) {
init(StoreProviderFactory.database(Group.class, Objects.requireNonNull(main)));
}
/**
* Get a group from the store.
*
* @param id The ID of a group
* @return The requested {@link Group}
*/
public static Optional get(String id) {
return provider.get("id", id);
}
/**
* Create a new group from the given configuration and add it to the store.
*
* @param config The group configuration
*/
public static void add(GroupConfig.Builder config) {
add(config.build());
}
/**
* Create a new group from the given configuration and add it to the store.
*
* @param config The group configuration
*/
public static void add(GroupConfig config) {
Objects.requireNonNull(config);
checkArgument(ValidationUtil.Config.valid(config) == ErrorCode.OK, "Invalid configuration");
checkArgument(ValidationUtil.Config.complete(config) == ErrorCode.OK, "Incomplete configuration");
add(new Group(config));
}
/**
* Add a group to the store.
*
* @param group The group to add
*/
public static void add(Group group) {
checkArgument(get(group.getGroupId()).isEmpty(), "ID conflict");
log.debug("Adding new group: {}", group.getGroupId());
provider.add(group);
}
/**
* Remove a group from the store.
*
* @param id The group's ID
*/
public static void remove(String id) {
log.debug("Deleting group {}", id);
get(id).ifPresent(provider::remove);
}
/**
* Get all groups that the given user owns or is a member of.
*
* @param user A user
* @return A list of the user's groups
*/
public static List getMembership(User user) {
try (Stream stream = provider.stream()) {
return stream.filter(group -> user.equals(group.getOwner()) || group.getMembers().contains(user))
.collect(Collectors.toList());
}
}
/**
* Get a list of all groups.
*
* @return A list of all groups in the store
*/
public static List getGroups() {
try (Stream stream = provider.stream()) {
return stream.collect(Collectors.toList());
}
}
/**
* Get a list of groups without an auth mechanism.
*
* @return A list of unauth groups
*/
public static List getUnauthGroups() {
try (Stream stream = provider.stream()) {
return stream.filter(group -> group.getKeys().size() == 0 && group.getPasswords().size() == 0)
.collect(Collectors.toList());
}
}
/**
* Get a list of groups with the given password.
*
* @param password The password
* @return A list of groups with the password
*/
public static List getByPassword(String password) {
try (Stream stream = provider.stream()) {
return stream.filter(
group -> group.getPasswords().stream().anyMatch(mech -> mech.getPassword().equals(password)))
.collect(Collectors.toList());
}
}
/**
* Change a group's configuration or statistics.
*
* @param id The ID of the group to modify
* @param delta The changes
* @return The outcome of the action
*/
public static Outcome delta(String id, ProtoGroup delta) {
var outcome = begin();
Group group = get(id).orElse(null);
if (group == null)
return failure(outcome, "Group not found");
ErrorCode error = group.merge(delta);
if (error != ErrorCode.OK)
return failure(outcome.setError(error));
return success(outcome);
}
private GroupStore() {
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy