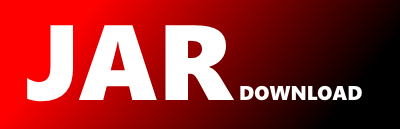
com.sangupta.jerry.jersey2.GsonJsonJerseyProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jerry-core Show documentation
Show all versions of jerry-core Show documentation
Common Java functionality for core functionality
package com.sangupta.jerry.jersey2;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.lang.annotation.Annotation;
import java.lang.reflect.Type;
import java.nio.charset.Charset;
import javax.ws.rs.Consumes;
import javax.ws.rs.Produces;
import javax.ws.rs.WebApplicationException;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.MultivaluedMap;
import javax.ws.rs.ext.Provider;
import org.glassfish.jersey.message.internal.AbstractMessageReaderWriterProvider;
import com.google.gson.FieldNamingPolicy;
import com.google.gson.Gson;
import com.sangupta.jerry.util.GsonUtils;
/**
* JSON serialization mechanism for Jersey. We use Google GSON library
* for serialization for its better performance and support.
*
* @author sangupta
* @since 2.0
*/
@Produces({ MediaType.APPLICATION_JSON })
@Consumes({ MediaType.APPLICATION_JSON })
@Provider
public class GsonJsonJerseyProvider extends AbstractMessageReaderWriterProvider
© 2015 - 2025 Weber Informatics LLC | Privacy Policy