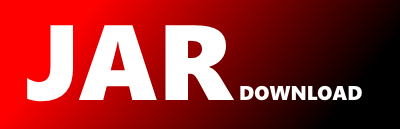
com.sangupta.jerry.util.ObjectUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jerry-core Show documentation
Show all versions of jerry-core Show documentation
Common Java functionality for core functionality
/**
*
* jerry - Common Java Functionality
* Copyright (c) 2012-2015, Sandeep Gupta
*
* http://sangupta.com/projects/jerry
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package com.sangupta.jerry.util;
/**
* Utility functions around {@link Object} instances.
*
* @author sangupta
*
*/
public class ObjectUtils {
/**
* Check if the object represents a primitive type of: byte
,
* char
, short
, int
,
* long
, float
, double
or
* boolean
.
*
* @param instance
* returns true
if the object is primitive type,
* false
otherwise. null
values return
* false
*
* @return true
if object is primitive, false
* otherwise or if object is null
*/
public static boolean isPrimitive(Object instance) {
if(instance == null) {
return false;
}
if(instance instanceof Integer) {
return true;
}
if(instance instanceof Byte) {
return true;
}
if(instance instanceof Short) {
return true;
}
if(instance instanceof Character) {
return true;
}
if(instance instanceof Long) {
return true;
}
if(instance instanceof Float) {
return true;
}
if(instance instanceof Double) {
return true;
}
if(instance instanceof Boolean) {
return true;
}
return false;
}
/**
* Check if given object array represents an array of primitives, i.e. in
* one of: int[]
, byte[]
, short[]
,
* char[]
, long[]
, float[]
,
* double[]
, boolean[]
*
* @param instance
* the object to be test
*
* @return true
if the object is a primitive aray,
* false
otherwise or if the object is
* null
*/
public static boolean isPrimitiveArray(Object instance) {
if(instance == null) {
return false;
}
if(instance instanceof int[]) {
return true;
}
if(instance instanceof byte[]) {
return true;
}
if(instance instanceof short[]) {
return true;
}
if(instance instanceof char[]) {
return true;
}
if(instance instanceof long[]) {
return true;
}
if(instance instanceof float[]) {
return true;
}
if(instance instanceof double[]) {
return true;
}
if(instance instanceof boolean[]) {
return true;
}
return false;
}
/**
* Convert the array into a {@link String} instance values separated by a
* COMMA.
*
* @param instance
* the primitive array instance that needs to be stringified
*
* @return the {@link String} representation, or null
*
* @throws IllegalArgumentException
* if the supplied instance is not a primitive array
*/
public static String stringifyPrimitiveArray(Object instance) {
if(instance == null) {
return null;
}
if(instance instanceof int[]) {
return StringUtils.stringifyArray((int[]) instance);
}
if(instance instanceof short[]) {
return StringUtils.stringifyArray((short[]) instance);
}
if(instance instanceof byte[]) {
return StringUtils.stringifyArray((byte[]) instance);
}
if(instance instanceof char[]) {
return StringUtils.stringifyArray((char[]) instance);
}
if(instance instanceof long[]) {
return StringUtils.stringifyArray((long[]) instance);
}
if(instance instanceof float[]) {
return StringUtils.stringifyArray((float[]) instance);
}
if(instance instanceof double[]) {
return StringUtils.stringifyArray((double[]) instance);
}
if(instance instanceof boolean[]) {
return StringUtils.stringifyArray((boolean[]) instance);
}
throw new IllegalArgumentException("Object is not a primitive array");
}
/**
* Check if given object array represents an array of boxed-primitives, i.e.
* in one of: Integer[]
, Byte[]
,
* Short[]
, Char[]
, Long[]
,
* Float[]
, Double[]
, Boolean[]
*
* @param instance
* the object to be test
*
* @return true
if the object is a boxed-primitive aray,
* false
otherwise or if the object is
* null
*/
public static boolean isBoxedPrimitiveArray(Object instance) {
if(instance == null) {
return false;
}
if(instance instanceof Integer[]) {
return true;
}
if(instance instanceof Byte[]) {
return true;
}
if(instance instanceof Short[]) {
return true;
}
if(instance instanceof Character[]) {
return true;
}
if(instance instanceof Long[]) {
return true;
}
if(instance instanceof Float[]) {
return true;
}
if(instance instanceof Double[]) {
return true;
}
if(instance instanceof Boolean[]) {
return true;
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy