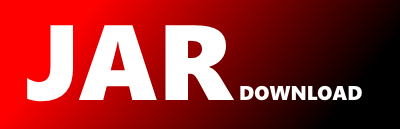
com.sangupta.jerry.util.PropertiesUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jerry-core Show documentation
Show all versions of jerry-core Show documentation
Common Java functionality for core functionality
The newest version!
/**
*
* jerry - Common Java Functionality
* Copyright (c) 2012-2017, Sandeep Gupta
*
* http://sangupta.com/projects/jerry-core
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package com.sangupta.jerry.util;
import java.util.HashMap;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
/**
* Utility methods to work with {@link Properties}.
*
* @author sangupta
*
*/
public abstract class PropertiesUtils {
/**
* Convert a given {@link Properties} instance to a corresponding
* {@link HashMap} instance. If the {@link Properties} instance is
* null
, a null
is returned back. If the instance
* is empty
, an empty
map is returned. Else, a
* {@link HashMap} with all keys is populated and returned.
*
* @param properties
* the {@link Properties} instance to be converted
*
* @return the corresponding {@link HashMap} instance, or null
*/
public static Map asMap(Properties properties) {
if(properties == null) {
return null;
}
Map map = new HashMap();
if(properties.isEmpty()) {
return map;
}
Set keys = properties.stringPropertyNames();
for(String key : keys) {
String value = properties.getProperty(key);
map.put(key, value);
}
return map;
}
/**
* Convert a given {@link Map} instance to a corresponding
* {@link Properties} instance
*
* @param map
* the map to convert
*
* @return the {@link Properties} instance thus created and populated
*/
public static Properties fromMap(Map map) {
if(map == null) {
return null;
}
Properties properties = new Properties();
if(map.isEmpty()) {
return properties;
}
Set keys = map.keySet();
for(String key : keys) {
properties.setProperty(key, map.get(key));
}
return properties;
}
/**
* Convert and return a {@link Boolean} value after reading from
* {@link Properties} the {@link String} value against the given
* key
. If no value exists a false
is returned.
*
* @param properties
* the {@link Properties} instance to read from
*
* @param key
* the key
to look for
*
* @return the {@link Boolean} value thus deciphered
*/
public static boolean getBooleanValue(Properties properties, String key) {
return getBooleanValue(properties, key, false);
}
/**
* Convert and return a {@link Boolean} value after reading from
* {@link Properties} the {@link String} value against the given
* key
. If no value exists the given defaultValue
* is returned.
*
* @param properties
* the {@link Properties} instance to read from
*
* @param key
* the key
to look for
*
* @param defaultValue
* the default value to return if the property cannot be found or
* the value specified for property is null
or empty
*
* @return the {@link Boolean} value thus deciphered
*/
public static boolean getBooleanValue(Properties properties, String key, boolean defaultValue) {
if(AssertUtils.isEmpty(properties)) {
return defaultValue;
}
String value = properties.getProperty(key);
return StringUtils.getBoolean(value, defaultValue);
}
/**
* Convert and return a {@link Short} value after reading from
* {@link Properties} the {@link String} value against the given
* key
. If no value exists the given defaultValue
* is returned.
*
* @param properties
* the {@link Properties} instance to read from
*
* @param key
* the key
to look for
*
* @param defaultValue
* the default value to return if the property cannot be found or
* the value specified for property is null
or empty
*
* @return the {@link Short} value thus deciphered
*/
public static short getShortValue(Properties properties, String key, short defaultValue) {
if(AssertUtils.isEmpty(properties)) {
return defaultValue;
}
String value = properties.getProperty(key);
return StringUtils.getShortValue(value, defaultValue);
}
/**
* Convert and return a {@link Integer} value after reading from
* {@link Properties} the {@link String} value against the given
* key
. If no value exists the given defaultValue
* is returned.
*
* @param properties
* the {@link Properties} instance to read from
*
* @param key
* the key
to look for
*
* @param defaultValue
* the default value to return if the property cannot be found or
* the value specified for property is null
or empty
*
* @return the {@link Integer} value thus deciphered
*/
public static int getIntValue(Properties properties, String key, int defaultValue) {
if(AssertUtils.isEmpty(properties)) {
return defaultValue;
}
String value = properties.getProperty(key);
return StringUtils.getIntValue(value, defaultValue);
}
/**
* Convert and return a {@link Long} value after reading from
* {@link Properties} the {@link String} value against the given
* key
. If no value exists the given defaultValue
* is returned.
*
* @param properties
* the {@link Properties} instance to read from
*
* @param key
* the key
to look for
*
* @param defaultValue
* the default value to return if the property cannot be found or
* the value specified for property is null
or empty
*
* @return the {@link Long} value thus deciphered
*/
public static long getLongValue(Properties properties, String key, long defaultValue) {
if(AssertUtils.isEmpty(properties)) {
return defaultValue;
}
String value = properties.getProperty(key);
return StringUtils.getLongValue(value, defaultValue);
}
/**
* Convert and return a {@link Float} value after reading from
* {@link Properties} the {@link String} value against the given
* key
. If no value exists the given defaultValue
* is returned.
*
* @param properties
* the {@link Properties} instance to read from
*
* @param key
* the key
to look for
*
* @param defaultValue
* the default value to return if the property cannot be found or
* the value specified for property is null
or empty
*
* @return the {@link Float} value thus deciphered
*/
public static float getFloatValue(Properties properties, String key, float defaultValue) {
if(AssertUtils.isEmpty(properties)) {
return defaultValue;
}
String value = properties.getProperty(key);
return StringUtils.getFloatValue(value, defaultValue);
}
/**
* Convert and return a {@link Double} value after reading from
* {@link Properties} the {@link String} value against the given
* key
. If no value exists the given defaultValue
* is returned.
*
* @param properties
* the {@link Properties} instance to read from
*
* @param key
* the key
to look for
*
* @param defaultValue
* the default value to return if the property cannot be found or
* the value specified for property is null
or empty
*
* @return the {@link Double} value thus deciphered
*/
public static double getDoubleValue(Properties properties, String key, double defaultValue) {
if(AssertUtils.isEmpty(properties)) {
return defaultValue;
}
String value = properties.getProperty(key);
return StringUtils.getDoubleValue(value, defaultValue);
}
/**
* Converts the {@link Object} value into a string representation taking
* care of the primitives, primitive arrays, boxed-primitive arrays, string
* arrays and more.
*
* @param value
* the {@link Object} instance that needs to be converted to
* {@link String} representation
*
* @return the {@link String} representation
*/
public static String getPropertyAsString(Object value) {
if(value == null) {
return null;
}
if(ObjectUtils.isPrimitive(value)) {
return value.toString();
}
if(value instanceof String) {
return (String) value;
}
if(ObjectUtils.isPrimitiveArray(value)) {
return ObjectUtils.stringifyPrimitiveArray(value);
}
if(ObjectUtils.isBoxedPrimitiveArray(value)) {
return StringUtils.stringifyArray((Object[]) value);
}
if(value instanceof String[]) {
// convert string array to string
return StringUtils.stringifyArray((String[]) value);
}
return value.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy