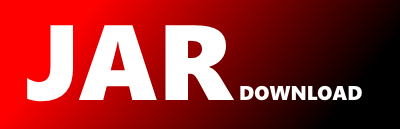
org.apache.olingo.client.api.serialization.ODataBinder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-v4-lib Show documentation
Show all versions of odata-v4-lib Show documentation
Repackaged OData V4 Olingo Library
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.olingo.client.api.serialization;
import org.apache.olingo.client.api.data.ResWrap;
import org.apache.olingo.client.api.data.ServiceDocument;
import org.apache.olingo.commons.api.data.Delta;
import org.apache.olingo.commons.api.data.Entity;
import org.apache.olingo.commons.api.data.EntityCollection;
import org.apache.olingo.commons.api.data.Link;
import org.apache.olingo.commons.api.data.Property;
import org.apache.olingo.client.api.domain.ClientDelta;
import org.apache.olingo.client.api.domain.ClientEntity;
import org.apache.olingo.client.api.domain.ClientEntitySet;
import org.apache.olingo.client.api.domain.ClientLink;
import org.apache.olingo.client.api.domain.ClientProperty;
import org.apache.olingo.client.api.domain.ClientServiceDocument;
public interface ODataBinder {
/**
* Gets a EntitySet from the given OData entity set.
*
* @param entitySet OData entity set.
* @return {@link EntityCollection} object.
*/
EntityCollection getEntitySet(ClientEntitySet entitySet);
/**
* Gets an Entity from the given OData entity.
*
* @param entity OData entity.
* @return {@link Entity} object.
*/
Entity getEntity(ClientEntity entity);
/**
* Gets a Link from the given OData link.
*
* @param link OData link.
* @return Link object.
*/
Link getLink(ClientLink link);
/**
* Gets a Property from the given OData property.
*
* @param property OData property.
* @return Property object.
*/
Property getProperty(ClientProperty property);
/**
* Adds the given property to the given entity.
*
* @param entity OData entity.
* @param property OData property.
* @return whether add was successful or not.
*/
boolean add(ClientEntity entity, ClientProperty property);
/**
* Gets ODataServiceDocument from the given service document resource.
*
* @param resource service document resource.
* @return ODataServiceDocument object.
*/
ClientServiceDocument getODataServiceDocument(ServiceDocument resource);
/**
* Gets ODataEntitySet from the given entity set resource.
*
* @param resource entity set resource.
* @return {@link ClientEntitySet} object.
*/
ClientEntitySet getODataEntitySet(ResWrap resource);
/**
* Gets ODataEntity from the given entity resource.
*
* @param resource entity resource.
* @return {@link ClientEntity} object.
*/
ClientEntity getODataEntity(ResWrap resource);
/**
* Gets an ODataProperty from the given property resource.
*
* @param resource property resource.
* @return {@link ClientProperty} object.
*/
ClientProperty getODataProperty(ResWrap resource);
ClientDelta getODataDelta(ResWrap resource);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy