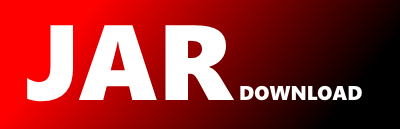
com.sap.cds.adapter.odata.v2.query.ExpressionToCqnVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cds-adapter-odata-v2 Show documentation
Show all versions of cds-adapter-odata-v2 Show documentation
OData V2 adapter for CDS Services Java
/**************************************************************************
* (C) 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
**************************************************************************/
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.sap.cds.adapter.odata.v2.query;
import static com.sap.cds.ql.CQL.func;
import static com.sap.cds.ql.CQL.val;
import java.util.ArrayList;
import java.util.List;
import java.util.Locale;
import org.apache.olingo.odata2.api.edm.EdmLiteral;
import org.apache.olingo.odata2.api.edm.EdmTyped;
import org.apache.olingo.odata2.api.uri.expression.BinaryExpression;
import org.apache.olingo.odata2.api.uri.expression.BinaryOperator;
import org.apache.olingo.odata2.api.uri.expression.ExpressionVisitor;
import org.apache.olingo.odata2.api.uri.expression.FilterExpression;
import org.apache.olingo.odata2.api.uri.expression.LiteralExpression;
import org.apache.olingo.odata2.api.uri.expression.MemberExpression;
import org.apache.olingo.odata2.api.uri.expression.MethodExpression;
import org.apache.olingo.odata2.api.uri.expression.MethodOperator;
import org.apache.olingo.odata2.api.uri.expression.OrderByExpression;
import org.apache.olingo.odata2.api.uri.expression.OrderExpression;
import org.apache.olingo.odata2.api.uri.expression.PropertyExpression;
import org.apache.olingo.odata2.api.uri.expression.SortOrder;
import org.apache.olingo.odata2.api.uri.expression.UnaryExpression;
import org.apache.olingo.odata2.api.uri.expression.UnaryOperator;
import org.apache.olingo.odata2.core.edm.EdmNull;
import com.sap.cds.adapter.odata.v2.utils.TypeConverterUtils;
import com.sap.cds.impl.builder.model.CqnNull;
import com.sap.cds.ql.CQL;
import com.sap.cds.ql.ElementRef;
import com.sap.cds.ql.Predicate;
import com.sap.cds.ql.Value;
import com.sap.cds.ql.cqn.CqnElementRef;
import com.sap.cds.ql.cqn.CqnReference.Segment;
import com.sap.cds.services.ErrorStatuses;
import com.sap.cds.services.utils.CdsErrorStatuses;
import com.sap.cds.services.utils.ErrorStatusException;
public class ExpressionToCqnVisitor implements ExpressionVisitor {
private static final String NULL_KEYWORD = "null";
private boolean caseInsensitive = false;
public ExpressionToCqnVisitor(boolean caseInsensitive) {
this.caseInsensitive = caseInsensitive;
}
@Override
@SuppressWarnings({ "unchecked", "rawtypes" })
public Object visitBinary(BinaryExpression binaryExpression, BinaryOperator operator, Object left, Object right) {
switch (operator) {
case AND:
return ((Predicate) left).and((Predicate) right);
case OR:
return ((Predicate) left).or((Predicate) right);
case EQ:
if (left instanceof Predicate lhs && right instanceof Predicate rhs) {
Predicate bothTrue = lhs.and(rhs);
Predicate bothFalse = lhs.not().and(rhs.not());
return bothTrue.or(bothFalse);
}
return ((Value) left).is((Value) right);
case NE:
if (left instanceof Predicate lhs && right instanceof Predicate rhs) {
Predicate eitherTrue = lhs.or(rhs);
Predicate eitherFalse = lhs.not().or(rhs.not());
return eitherTrue.and(eitherFalse);
}
return ((Value) left).isNot((Value) right);
case GE:
return ((Value) left).ge(right);
case GT:
return ((Value) left).gt(right);
case LE:
return ((Value) left).le(right);
case LT:
return ((Value) left).lt(right);
case ADD:
return ((Value) left).plus((Value) right);
case SUB:
return ((Value) left).minus((Value) right);
case MUL:
return ((Value) left).times((Value) right);
case DIV:
return ((Value) left).dividedBy((Value) right);
default:
throw new ErrorStatusException(CdsErrorStatuses.UNSUPPORTED_OPERATOR, operator);
}
}
@Override
public Object visitUnary(UnaryExpression unaryExpression, UnaryOperator operator, Object operand) {
switch (operator) {
case NOT:
return ((Predicate) operand).not();
default:
throw new ErrorStatusException(ErrorStatuses.NOT_IMPLEMENTED);
}
}
@Override
@SuppressWarnings("unchecked")
public Object visitMethod(MethodExpression methodExpression, MethodOperator methodCall, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy