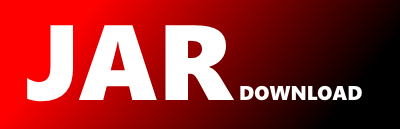
com.sap.cds.adapter.odata.v2.utils.UriInfoUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cds-adapter-odata-v2 Show documentation
Show all versions of cds-adapter-odata-v2 Show documentation
OData V2 adapter for CDS Services Java
/**************************************************************************
* (C) 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
**************************************************************************/
package com.sap.cds.adapter.odata.v2.utils;
import java.util.List;
import java.util.Objects;
import org.apache.olingo.odata2.api.edm.EdmEntityType;
import org.apache.olingo.odata2.api.edm.EdmException;
import org.apache.olingo.odata2.api.edm.EdmProperty;
import org.apache.olingo.odata2.api.edm.EdmType;
import org.apache.olingo.odata2.api.uri.UriInfo;
import com.sap.cds.adapter.odata.v2.CdsRequestGlobals;
import com.sap.cds.adapter.odata.v2.processors.request.CdsODataRequest;
import com.sap.cds.services.ServiceException;
import com.sap.cds.services.utils.CdsErrorStatuses;
import com.sap.cds.services.utils.ErrorStatusException;
public class UriInfoUtils {
private static final String PARAMETERS = "Parameters"; // suffix for parameter entity type
private static final String TYPE = "Type"; // suffix for entity type of Set navigation
private static final String SET = "Set"; // navigation property on Parameter entity type
private final CdsRequestGlobals globals;
public UriInfoUtils(CdsRequestGlobals globals) {
this.globals = Objects.requireNonNull(globals, "globals");
}
public static EdmProperty getSimpleProperty(UriInfo uriInfo) {
List propertyPath = uriInfo.getPropertyPath();
if (!propertyPath.isEmpty()) {
return propertyPath.get(propertyPath.size() - 1);
}
throw new ServiceException("No property path defined");
}
public static EdmProperty getSimpleProperty(CdsODataRequest cdsODataRequest) {
return getSimpleProperty(cdsODataRequest.getUriInfo());
}
public String getTargetEntityName(UriInfo uriInfo) {
try {
return getCdsEntityName(uriInfo.getTargetEntitySet().getEntityType());
} catch (EdmException e) {
throw new ServiceException(e);
}
}
private static String concat(String ns, String name) {
return ns + "." + name;
}
public String getFullQualifiedName(EdmType entityType) {
try {
String namespace = entityType.getNamespace();
String name = entityType.getName();
String fqName = concat(namespace, name);
String cdsName = globals.getCdsEntityNames().get(fqName);
if (cdsName != null) {
fqName = cdsName;
}
return fqName;
} catch (EdmException e) {
throw new ServiceException(e);
}
}
public boolean isParametersEntityType(EdmEntityType entityType) {
String name = getFullQualifiedName(entityType);
// potential [CdsView]Parameters type
if (name.endsWith(PARAMETERS)) {
try {
// has Set navigation
if (entityType.getNavigationPropertyNames().contains(SET)) {
String setName = getFullQualifiedName(entityType.getProperty(SET).getType());
// Set type is named [CdsView]Type and both refer to the same [CdsView]
if(setName.endsWith(TYPE) && name.startsWith(setName.substring(0, setName.length() - TYPE.length()))) {
return true;
}
}
} catch (EdmException e) {
throw new ServiceException(e);
}
}
return false;
}
public boolean isSetEntityType(EdmEntityType entityType) {
String name = getFullQualifiedName(entityType);
// potential [CdsView]Type type
if (name.endsWith(TYPE)) {
try {
// has Parameters navigation
if (entityType.getNavigationPropertyNames().contains(PARAMETERS)) {
String paramName = getFullQualifiedName(entityType.getProperty(PARAMETERS).getType());
// Parameters type is named [CdsView]Parameters and both refer to the same [CdsView]
if(paramName.endsWith(PARAMETERS) && name.startsWith(paramName.substring(0, paramName.length() - PARAMETERS.length()))) {
return true;
}
}
} catch (EdmException e) {
throw new ServiceException(e);
}
}
return false;
}
public String getCdsEntityName(EdmEntityType entityType) {
String name = getFullQualifiedName(entityType);
if(isSetEntityType(entityType)) {
return name.substring(0, name.length() - TYPE.length());
} else if (isParametersEntityType(entityType)) {
throw new ErrorStatusException(CdsErrorStatuses.INVALID_PARAMETERIZED_VIEW);
} else {
return name;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy