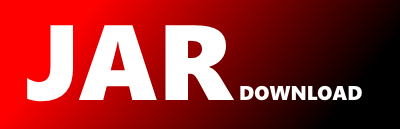
com.sap.cds.adapter.odata.v4.query.apply.HierarchySubsetConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cds-adapter-odata-v4 Show documentation
Show all versions of cds-adapter-odata-v4 Show documentation
OData V4 adapter for CDS Services Java
/**************************************************************************
* (C) 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
**************************************************************************/
package com.sap.cds.adapter.odata.v4.query.apply;
import java.util.List;
import org.apache.olingo.server.api.uri.queryoption.ApplyItem;
import org.apache.olingo.server.api.uri.queryoption.apply.Ancestors;
import org.apache.olingo.server.api.uri.queryoption.apply.Descendants;
import org.apache.olingo.server.api.uri.queryoption.apply.HierarchicalSubsetTransformation;
import com.sap.cds.adapter.odata.v4.query.ExpressionParser;
import com.sap.cds.ql.CQL;
import com.sap.cds.ql.cqn.CqnElementRef;
import com.sap.cds.ql.cqn.CqnStructuredTypeRef;
import com.sap.cds.ql.cqn.transformation.CqnAncestorsTransformation;
import com.sap.cds.ql.cqn.transformation.CqnTransformation;
import com.sap.cds.ql.impl.transformations.AncestorsTrafo;
import com.sap.cds.ql.impl.transformations.DescendantsTrafo;
public abstract class HierarchySubsetConverter {
public static CqnAncestorsTransformation ancestors(Ancestors ancestors, ExpressionParser expressionParser) {
ParamConverter converter = new ParamConverter(ancestors, expressionParser);
return AncestorsTrafo
.ancestors(converter.hierarchyReference(), converter.hierarchyQualifier(), converter.nodeProperty())
.distanceFromStart(converter.distinctFromStart()).keepStart(converter.keepStart())
.transformations(converter.transformations());
}
public static CqnTransformation descendants(Descendants descendants, ExpressionParser expressionParser) {
ParamConverter converter = new ParamConverter(descendants, expressionParser);
return DescendantsTrafo
.descendants(converter.hierarchyReference(), converter.hierarchyQualifier(), converter.nodeProperty())
.distanceFromStart(converter.distinctFromStart()).keepStart(converter.keepStart())
.transformations(converter.transformations());
}
private static class ParamConverter {
private ExpressionParser parser;
private HierarchicalSubsetTransformation transformation;
public ParamConverter(HierarchicalSubsetTransformation transformation, ExpressionParser expressionParser) {
this.transformation = transformation;
this.parser = expressionParser;
}
CqnStructuredTypeRef hierarchyReference() {
return parser.getTargetTypeRef(transformation.getHierarchyReference());
}
String hierarchyQualifier() {
return transformation.getHierarchyQualifier();
}
CqnElementRef nodeProperty() {
return CQL.get(parser.toSegmentList(transformation.getNodeProperty()));
}
List transformations() {
List applies = transformation.getTransformations();
List transformations;
ApplyHandler applyHandler = new ApplyHandler(applies, parser);
transformations = applyHandler.getTransformations(0);
return transformations;
}
int distinctFromStart() {
return transformation.getDistanceFromStart() != null ? transformation.getDistanceFromStart() : -1;
}
boolean keepStart() {
return transformation.isKeepStart() == Boolean.TRUE;// TODO check default
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy