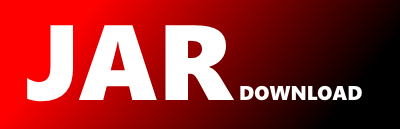
com.sap.cds.adapter.odata.v4.query.apply.TopLevelsConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cds-adapter-odata-v4 Show documentation
Show all versions of cds-adapter-odata-v4 Show documentation
OData V4 adapter for CDS Services Java
/**************************************************************************
* (C) 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
**************************************************************************/
package com.sap.cds.adapter.odata.v4.query.apply;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import org.apache.olingo.server.api.uri.UriParameter;
import org.apache.olingo.server.api.uri.queryoption.apply.CustomFunction;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.sap.cds.adapter.odata.v4.metadata.extension.CustomVocabularyCatalog;
import com.sap.cds.adapter.odata.v4.query.ExpressionParser;
import com.sap.cds.ql.CQL;
import com.sap.cds.ql.cqn.CqnElementRef;
import com.sap.cds.ql.cqn.CqnStructuredTypeRef;
import com.sap.cds.ql.cqn.transformation.CqnTopLevelsTransformation;
import com.sap.cds.ql.impl.transformations.TopLevelsTrafo;
public class TopLevelsConverter {
private static final String NAME = CustomVocabularyCatalog.COM_SAP_VOCABULARY_HIERARCHY + "."
+ CustomVocabularyCatalog.TOP_LEVELS;
private TopLevelsConverter() {
// empty
}
public static CqnTopLevelsTransformation of(CustomFunction custom, ExpressionParser expressionParser) {
ParamConverter converter = new ParamConverter(custom, expressionParser);
return TopLevelsTrafo.topLevels(converter.levels(), converter.expandLevels())
.hierarchy(converter.hierarchyReference(), converter.hierarchyQualifier(), converter.nodeProperty());
}
private static class ParamConverter {
private final ExpressionParser parser;
private final Map params;
private static final ObjectMapper mapper = new ObjectMapper();
public ParamConverter(CustomFunction transformation, ExpressionParser expressionParser) {
params = transformation.getParameters().stream().collect(Collectors.toMap(UriParameter::getName, p -> p));
this.parser = expressionParser;
}
CqnStructuredTypeRef hierarchyReference() {
UriParameter ref = params.get("HierarchyNodes");
return parser.getTargetTypeRef(ref.getExpression());
}
String hierarchyQualifier() {
return unquote(params.get("HierarchyQualifier").getText());
}
CqnElementRef nodeProperty() {
UriParameter node = params.get("NodeProperty");
return CQL.get(node.getText());
}
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy