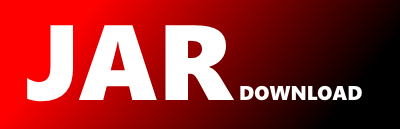
com.sap.cds.adapter.rest.utils.Json Maven / Gradle / Ivy
/**************************************************************************
* (C) 2019-2021 SAP SE or an SAP affiliate company. All rights reserved. *
**************************************************************************/
package com.sap.cds.adapter.rest.utils;
import java.io.Reader;
import java.util.Map;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
/**
* Helper class for JSON mappings that hides the concrete JSON open source library.
*/
public class Json {
private final static ObjectMapper mapper = new ObjectMapper();
private JsonNode json;
private Json(JsonNode jsonNode) {
this.json = jsonNode;
}
public static Json fromReader(Reader reader) throws JsonException {
try {
return new Json( mapper.readTree(reader) );
} catch (Exception e) { //NOSONAR
throw new JsonException("Failed to parse JSON data from Reader", e);
}
}
public static Json fromString(String jsonData) throws JsonException {
try {
return new Json( mapper.readTree(jsonData) );
} catch (Exception e) { //NOSONAR
throw new JsonException("Failed to parse JSON data from '" + jsonData + "'", e);
}
}
public static Json fromMap(Map entry) {
return new Json(mapper.valueToTree(entry));
}
@SuppressWarnings("unchecked")
public Map asMap() throws JsonException {
if (json.isArray()) {
throw new IllegalStateException("JSON is not an JSON object");
}
try {
return mapper.treeToValue(this.json, Map.class);
} catch (JsonProcessingException e) {
throw new JsonException("Failed to convert JSON to map", e);
}
}
public String asString() {
return json.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy