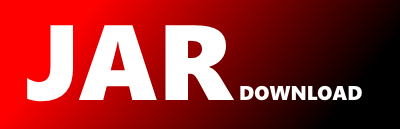
com.sap.cds.feature.messaging.mq.client.MessageQueuingManagmentClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cds-feature-message-queuing Show documentation
Show all versions of cds-feature-message-queuing Show documentation
Message Queuing feature for CDS Services Java
/**************************************************************************
* (C) 2019-2021 SAP SE or an SAP affiliate company. All rights reserved. *
**************************************************************************/
package com.sap.cds.feature.messaging.mq.client;
import java.io.IOException;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.Map;
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.sap.cds.integration.cloudsdk.rest.client.JsonRestClient;
import com.sap.cds.services.environment.ServiceBinding;
import com.sap.cloud.sdk.cloudplatform.connectivity.OnBehalfOf;
/**
* Implementation of the enterprise messaging management API.
*/
public class MessageQueuingManagmentClient extends JsonRestClient {
private static final String API_BASE = "/v1/management";
private static final String API_QUEUE = API_BASE + "/queues/%s";
private static final String API_SUBSCRIBE = API_BASE + "/queues/%s/subscriptions/topics/%s";
public MessageQueuingManagmentClient(ServiceBinding binding) {
super(getManagementApiUrlFromBinding(binding), binding, OnBehalfOf.TECHNICAL_USER_PROVIDER);
}
@SuppressWarnings("unchecked")
private static String getManagementApiUrlFromBinding(ServiceBinding binding) {
return (String) ((Map) binding.getCredentials().get("management")).get("url");
}
public void removeQueue(String name) throws IOException {
String encName = URLEncoder.encode(name, StandardCharsets.UTF_8.toString());
deleteRequest(String.format(API_QUEUE, encName));
}
public void createQueue(String name, Map properties) throws IOException {
ObjectNode data = mapper.createObjectNode();
properties.forEach((key, value) -> {
data.put(key, value);
});
String encName = URLEncoder.encode(name, StandardCharsets.UTF_8.toString());
putRequest(String.format(API_QUEUE, encName), data);
}
public void createQueueSubscription(String queue, String topic) throws IOException {
String encQueue = URLEncoder.encode(queue, StandardCharsets.UTF_8.toString());
String encTopic = URLEncoder.encode(topic, StandardCharsets.UTF_8.toString());
putRequest(String.format(API_SUBSCRIBE, encQueue, encTopic), null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy