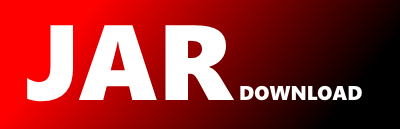
com.sap.cds.feature.mt.RoutingDataSourceFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cds-feature-mt Show documentation
Show all versions of cds-feature-mt Show documentation
Multi tenancy feature for CDS Services Java
/**************************************************************************
* (C) 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
**************************************************************************/
package com.sap.cds.feature.mt;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Map.Entry;
import javax.sql.DataSource;
import com.sap.cds.services.datasource.DataSourceFactory;
import com.sap.cds.services.environment.CdsProperties.MultiTenancy;
import com.sap.cds.services.request.RequestContext;
import com.sap.cds.services.runtime.CdsRuntime;
import com.sap.cds.services.runtime.CdsRuntimeAware;
import com.sap.cds.services.utils.CdsErrorStatuses;
import com.sap.cds.services.utils.ErrorStatusException;
import com.sap.cds.services.utils.datasource.DataSourceUtils;
import com.sap.cds.services.utils.datasource.DataSourceUtils.PoolType;
import com.sap.cloud.mt.runtime.DataPoolSettings;
import com.sap.cloud.mt.runtime.DataPoolSettings.ConnectionPoolType;
import com.sap.cloud.mt.runtime.DataSourceLookup;
import com.sap.cloud.mt.runtime.DataSourceLookupBuilder;
import com.sap.cloud.mt.runtime.EnvironmentAccess;
import com.sap.cloud.mt.runtime.IdentityZoneDeterminer;
import com.sap.cloud.mt.runtime.TenantAwareDataSource;
import com.sap.cloud.mt.runtime.TenantProvider;
import com.sap.cloud.mt.subscription.InstanceLifecycleManager;
/**
* Provider of a tenant dependent data source.
*/
public class RoutingDataSourceFactory implements DataSourceFactory, CdsRuntimeAware {
private static final String DATA_SOURCE_SECTION_MT = "cds.multitenancy.datasource.";
private CdsRuntime runtime;
private MtUtils mtUtils;
@Override
public void setCdsRuntime(CdsRuntime runtime) {
this.runtime = runtime;
this.mtUtils = new MtUtils(runtime);
}
@Override
public Map create() {
Map dataSources = new HashMap<>();
for (Entry entry : mtUtils.createInstanceLifecycleManagers().entrySet()) {
dataSources.put(entry.getKey(), createRoutingDataSource(entry.getKey(), entry.getValue()));
}
return dataSources;
}
private DataSource createRoutingDataSource(String name, InstanceLifecycleManager ilm) {
Map parameterConfig = createParameterConfig(name);
MultiTenancy multiTenancy = runtime.getEnvironment().getCdsProperties().getMultiTenancy();
DataSourceLookup lookup = DataSourceLookupBuilder.create()
.instanceLifecycleManager(ilm)
.environmentAccess(new EnvironmentAccess() {
@Override
public T getProperty(String key, Class clazz) {
return runtime.getEnvironment().getProperty(key, clazz, null);
}
@Override
@SuppressWarnings("unchecked")
public Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy