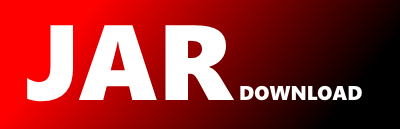
com.sap.cds.feature.mt.SmsClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cds-feature-mt Show documentation
Show all versions of cds-feature-mt Show documentation
Multi tenancy feature for CDS Services Java
/**************************************************************************
* (C) 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
**************************************************************************/
package com.sap.cds.feature.mt;
import java.io.IOException;
import java.net.URI;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.node.BooleanNode;
import com.sap.cloud.environment.servicebinding.api.ServiceIdentifier;
import com.sap.cloud.sdk.cloudplatform.connectivity.DefaultOAuth2PropertySupplier;
import com.sap.cloud.sdk.cloudplatform.connectivity.OAuth2ServiceBindingDestinationLoader;
import com.sap.cloud.sdk.cloudplatform.connectivity.ServiceBindingDestinationOptions;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.node.ArrayNode;
import com.google.common.base.Objects;
import com.sap.cds.integration.cloudsdk.rest.client.JsonRestClient;
import com.sap.cds.services.runtime.CdsRuntime;
import com.sap.cds.services.utils.environment.ServiceBindingUtils;
import com.sap.cloud.environment.servicebinding.api.ServiceBinding;
import com.sap.cloud.sdk.cloudplatform.connectivity.OnBehalfOf;
/**
* Implementation of the SMS REST client.
*/
public class SmsClient extends JsonRestClient {
private static final Logger logger = LoggerFactory.getLogger(SmsClient.class);
private static final int PAGE_SIZE = 500;
private static final String SMS = "subscription-manager";
public static final String BINDING_URL_KEY = "subscription_manager_url";
public static final String SUBSCRIPTIONS_PATH = "/subscription-manager/v1/subscriptions";
public static final String SUBSCRIPTION_STATE = "subscriptionState";
public static Optional findBinding(CdsRuntime runtime) {
return runtime.getEnvironment().getServiceBindings().filter(b -> ServiceBindingUtils.matches(b, null, SMS)).findFirst();
}
static {
OAuth2ServiceBindingDestinationLoader.registerPropertySupplier(
ServiceIdentifier.of(SMS),
SmsClient.OAuth2Supplier::new);
}
private static class OAuth2Supplier extends DefaultOAuth2PropertySupplier {
public OAuth2Supplier(ServiceBindingDestinationOptions options) {
super(options, Collections.emptyList());
}
@Override
public URI getServiceUri() {
return getCredentialOrThrow(URI.class, BINDING_URL_KEY);
}
}
public SmsClient(ServiceBinding binding) {
super(ServiceBindingDestinationOptions.forService(binding).onBehalfOf(OnBehalfOf.TECHNICAL_USER_PROVIDER).build());
}
/**
* Retrieves the tenant info of all subscribed tenants
*
* @return tenants info
*
* @throws IOException throws when any connection problems occur
*/
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy