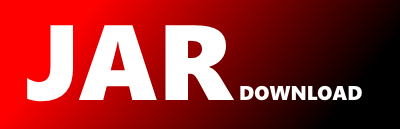
com.sap.cds.services.mt.SmsSubscriber Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cds-feature-mt Show documentation
Show all versions of cds-feature-mt Show documentation
Multi tenancy feature for CDS Services Java
/**************************************************************************
* (C) 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
**************************************************************************/
package com.sap.cds.services.mt;
import java.util.Map;
import com.sap.cds.CdsData;
import com.sap.cds.Struct;
import com.sap.cds.ql.CdsName;
/**
* An SMS (Subscription Manager Service) subscriber.
*/
public interface SmsSubscriber extends CdsData {
String APP_TID = "app_tid";
static SmsSubscriber create() {
return Struct.create(SmsSubscriber.class);
}
static SmsSubscriber create(Map attributes) {
return Struct.access(attributes).as(SmsSubscriber.class);
}
/**
* Gets the subaccount id.
*
* @return the subaccount id
*/
String getSubaccountId();
/**
* Sets the subaccount id.
*
* @param id the subaccount id
*/
void setSubaccountId(String id);
/**
* Gets the subaccount subdomain.
*
* @return the subdomain
*/
String getSubaccountSubdomain();
/**
* Sets the subaccount subdomain.
*
* @param domain the subdomain
*/
void setSubaccountSubdomain(String domain);
/**
* Gets the global account id.
*
* @return the account id
*/
String getGlobalAccountId();
/**
* Sets the global account id.
*
* @param id the account id
*/
void setGlobalAccountId(String id);
/**
* Gets the subscription GUID.
*
* @return the guid
*/
String getSubscriptionGUID();
/**
* Sets the subscription GUID.
*
* @param guid the guid
*/
void setSubscriptionGUID(String guid);
/**
* Gets the license type.
*
* @return the license type
*/
String getLicenseType();
/**
* Sets the license type.
*
* @param licenceType the license type
*/
void setLicenseType(String licenceType);
/**
* Gets the user id.
*
* @return the user id
*/
String getUserId();
/**
* Sets the user id.
*
* @param id the user id
*/
void setUserId(String id);
/**
* Gets the zone id.
*
* @return the zone id
*/
String getZoneId();
/**
* Sets the zone id.
*
* @param id the zone id
*/
void setZoneId(String id);
/**
* Gets the application tenant id.
*
* @return the app tenant id
*/
@CdsName(APP_TID)
String getAppTid();
/**
* Sets the application tenant id.
*
* @param id the app tenant id
*/
@CdsName(APP_TID)
void setAppTid(String id);
/**
* Gets the zone name.
*
* @return the zone name
*/
String getZoneName();
/**
* Sets the zone name.
*
* @param name the zone name
*/
void setZoneName(String name);
/**
* Gets the tenant host.
*
* @return the tenant host
*/
String getTenantHost();
/**
* Sets the tenant host.
*
* @param host the tenant host
*/
void setTenantHost(String host);
SmsUserInfo getUserInfo();
void setUserInfo(SmsUserInfo userInfo);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy