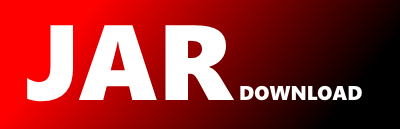
com.sap.cds.CdsDataStore Maven / Gradle / Ivy
/*******************************************************************
* © 2019 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds;
import static java.util.Collections.emptyMap;
import java.util.Map;
import java.util.stream.Stream;
import com.google.common.annotations.Beta;
import com.sap.cds.ql.CQL;
import com.sap.cds.ql.Delete;
import com.sap.cds.ql.Insert;
import com.sap.cds.ql.Select;
import com.sap.cds.ql.Update;
import com.sap.cds.ql.Upsert;
import com.sap.cds.ql.cqn.CqnDelete;
import com.sap.cds.ql.cqn.CqnInsert;
import com.sap.cds.ql.cqn.CqnSelect;
import com.sap.cds.ql.cqn.CqnUpdate;
import com.sap.cds.ql.cqn.CqnUpsert;
import com.sap.cds.reflect.CdsEntity;
/**
* The CdsDataStore is used to interact with a data source. It allows to execute
* CDS QL {@link Select queries}, as well as {@link Insert insert},
* {@link Upsert upsert}, {@link Update update} and {@link Delete delete}
* statements.
*/
public interface CdsDataStore {
/**
* Executes a {@link CqnSelect} statement with optional positional values for
* indexed parameters, see {@link CQL#param(int)}.
*
* @param select the CQN select statement to be executed
* @param paramValues the positional parameter values
* @return the {@link Result} of the query
* @throws CdsLockTimeoutException if the statement uses {@link Select#lock()
* locking} but a lock can't be acquired
*/
Result execute(CqnSelect select, Object... paramValues);
/**
* Executes a {@link CqnSelect} statement with values for named parameters, see
* {@link CQL#param(String)}.
*
* @param select the CQN select statement to be executed
* @param namedValues the named parameter values
* @return the {@link Result} of the query
* @throws CdsLockTimeoutException if the statement uses {@link Select#lock()
* locking} but a lock can't acquired
*/
Result execute(CqnSelect select, Map namedValues);
/**
* Executes a {@link CqnSelect} statement as batch with the given named
* parameter values. Named parameters are required either in the where clause,
* or in one infix filter of the from clause.
*
* If the number of entries in valueSets is larger than the default batch size,
* the select statement is executed multiple times and the result sets are
* combined, in this case order by clauses are not supported and an exception is
* thrown.
*
* @param select the CQN select statement to be executed
* @param valueSets the named parameter value sets
* @return the {@link Result} of the batch select
* @throws CdsLockTimeoutException if the statement uses {@link Select#lock()
* locking} but a lock can't acquired
*/
@Beta
default Result execute(CqnSelect select, Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy