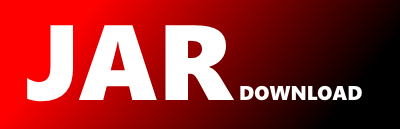
com.sap.cds.CdsList Maven / Gradle / Ivy
/*******************************************************************
* © 2022 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import com.sap.cds.ql.cqn.CqnInsert;
import com.sap.cds.ql.cqn.CqnUpdate;
import com.sap.cds.ql.cqn.CqnUpsert;
/**
* A list of data to be used in {@link CqnInsert Insert}, {@link CqnUpdate
* Update} and {@link CqnUpsert Upsert} statements.
*
* In deep updates and upserts, nested lists can be marked as a
* {@link CdsList#delta() delta representation}, which may contain entities that
* are {@link CdsData#forRemoval() marked for removal}.
*/
public interface CdsList> extends List, JSONizable {
Factory factory = Cds4jServiceLoader.load(Factory.class);
/**
* Creates a new instance of {@link CdsList}.
*
* @return an empty {@link CdsList}
*/
static > CdsList create() {
return factory.create();
}
/**
* Returns a {@link CdsList} with the given entries.
*
* @param entries
* the list entries
*
* @return a {@link CdsList} with the given entries
*/
@SafeVarargs
static > CdsList create(T... entries) {
return factory.create(Arrays.asList(entries));
}
/**
* Returns a delta {@link CdsList} with the given entries.
*
* @param deltaEntries
* the delta list entries
*
* @return a delta {@link CdsList} with the given entries
*/
@SafeVarargs
static > CdsList delta(T... deltaEntries) {
return create(deltaEntries).delta();
}
/**
* Returns a {@link CdsList} backed by the given list.
*
* @param backingList
* the backing list
*
* @return a {@link CdsList} backed by backingList
*/
static > CdsList create(List backingList) {
return factory.create(backingList);
}
/**
* Marks this list as a delta representation for a nested collection in
* deep {@link CqnUpdate update} or {@link CqnUpsert upsert} data.
*
* @return this list instance
*/
default CdsList delta() {
setDelta(true);
return this;
}
/**
* Marks or unmarks this list as a delta representation for a
* nested collection in deep {@link CqnUpdate update} or {@link CqnUpsert
* upsert} data.
*
* @param delta
* true to mark as delta, false to unmark
*/
void setDelta(boolean delta);
/**
* Indicates if this list is marked as a delta representation for a
* nested collection in deep {@link CqnUpdate update} or {@link CqnUpsert
* upsert} data
*
* @return true if this instance if marked as delta
*/
boolean isDelta();
public interface Factory {
> CdsList create();
> CdsList create(List list);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy