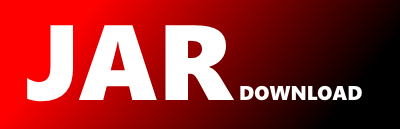
com.sap.cds.NotNullConstraintException Maven / Gradle / Ivy
/*******************************************************************
* © 2020 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds;
import static java.util.Collections.unmodifiableList;
import java.io.Serial;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import com.sap.cds.ql.CdsDataException;
import com.sap.cds.reflect.CdsElement;
/**
* Thrown to indicate that a NOT NULL constraint has been violated
* during an attempt to insert or update a CdsEntity.
*/
public class NotNullConstraintException extends CdsDataException {
@Serial
private static final long serialVersionUID = 1L;
private final transient List nonNullableElements;
public NotNullConstraintException(List nonNullableElements, Exception ex) {
super("Constraint violated: %s must not be NULL.".formatted(stringify(nonNullableElements)), ex);
this.nonNullableElements = unmodifiableList(nonNullableElements);
}
/**
* Get list of qualified element names which should not be null
*
* @return list of qualified element names
*/
public List getElementNames() {
return names(nonNullableElements).collect(Collectors.toList());
}
/**
* Get the qualified name of the entity
*
* @return qualified name of the entity
*/
public String getEntityName() {
return nonNullableElements.get(0).getDeclaringType().getQualifiedName();
}
private static String stringify(List nonNullableElements) {
return names(nonNullableElements).map(e -> "'" + e + "'").collect(Collectors.joining(", "));
}
private static Stream names(List nonNullableElements) {
return nonNullableElements.stream().map(CdsElement::getQualifiedName);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy