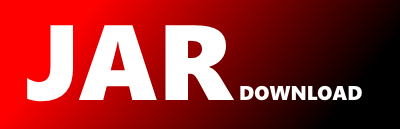
com.sap.cds.Struct Maven / Gradle / Ivy
/*******************************************************************
* © 2019 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds;
import static java.util.Collections.unmodifiableMap;
import java.util.HashMap;
import java.util.Map;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
/**
* Struct provides static helper methods to access data given as {@code Map} via
* an accessor interface.
*/
public final class Struct {
private static final ProxyFactory proxyFactory = Cds4jServiceLoader.load(ProxyFactory.class);
private Struct() {
}
/**
* Creates a data container for the given accessor interface.
*
* @param the return type
* @param type the accessor interface
* @return a typed data container
*/
public static T create(Class type) {
return proxyFactory.proxy(new HashMap<>(), type);
}
/**
* Provides typed access on the given data map.
*
* @param data the data map to access
* @return a factory, that provides typed access on the underlying data
*/
public static MapViewFactory access(Map data) {
return new MapViewFactory(data);
}
/**
* Provides typed stream access on the given data maps.
*
* @param data the data maps to be streamed
* @return a factory, that provides typed access on the underlying data
*/
public static IterableViewFactory stream(Iterable extends Map> data) {
return new IterableViewFactory(data);
}
public interface ProxyFactory {
T proxy(Map data, Class type);
}
public static final class MapViewFactory {
private final Map data;
private MapViewFactory(Map data) {
this.data = data; // NOSONAR
}
/**
* Provides typed access on the underlying data map.
*
* @param the return type
* @param view the accessor interface
* @return a typed writable view on the underlying data map
*/
public T as(Class view) {
return proxyFactory.proxy(data, view);
}
/**
* Provides typed read-only access on the underlying data map.
*
* @param the return type
* @param view the accessor interface
* @return a typed read-only view on the underlying data map
*/
public T asReadOnly(Class view) {
return proxyFactory.proxy(unmodifiableMap(data), view);
}
}
public static final class IterableViewFactory {
private final Iterable extends Map> data;
private IterableViewFactory(Iterable extends Map> data) {
this.data = data;
}
/**
* Provides typed stream access on the underlying data maps.
*
* @param the type of the returned {@code Stream}
* @param view the accessor interface
* @return a typed {@code Stream} of the underlying data maps
*/
public Stream as(Class view) {
return StreamSupport.stream(data.spliterator(), false).map(d -> proxyFactory.proxy(d, view));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy