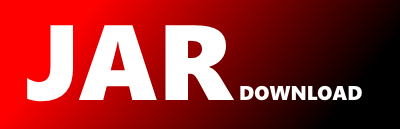
com.sap.cds.ql.CqnBuilder Maven / Gradle / Ivy
/************************************************************************
* © 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
************************************************************************/
package com.sap.cds.ql;
import java.time.Instant;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
import java.util.function.UnaryOperator;
import java.util.stream.Collector;
import com.sap.cds.ql.cqn.CqnArithmeticExpression;
import com.sap.cds.ql.cqn.CqnComparisonPredicate;
import com.sap.cds.ql.cqn.CqnConnectivePredicate.Operator;
import com.sap.cds.ql.cqn.CqnContainmentTest;
import com.sap.cds.ql.cqn.CqnElementRef;
import com.sap.cds.ql.cqn.CqnExpand;
import com.sap.cds.ql.cqn.CqnListValue;
import com.sap.cds.ql.cqn.CqnMatchPredicate.Quantifier;
import com.sap.cds.ql.cqn.CqnPredicate;
import com.sap.cds.ql.cqn.CqnReference.Segment;
import com.sap.cds.ql.cqn.CqnSelect;
import com.sap.cds.ql.cqn.CqnSortSpecification;
import com.sap.cds.ql.cqn.CqnSortSpecification.Order;
import com.sap.cds.ql.cqn.CqnSource;
import com.sap.cds.ql.cqn.CqnStatement;
import com.sap.cds.ql.cqn.CqnStructuredTypeRef;
import com.sap.cds.ql.cqn.CqnValue;
import com.sap.cds.ql.cqn.CqnVector;
import com.sap.cds.ql.cqn.Modifier;
import com.sap.cds.reflect.CdsEntity;
/**
* Service Provider Interface
*/
public interface CqnBuilder {
static CqnBuilder instance() {
return CDS.QL.builder;
}
Select> select(String entityName);
Select> select(String entityName, UnaryOperator> path);
Select> select(CqnSource source);
Insert insert(String entityName, UnaryOperator> path);
Upsert upsert(String entityName, UnaryOperator> path);
Update> update(String entityName, UnaryOperator> path);
Delete> delete(String entityName, UnaryOperator> path);
Insert insert(CqnStructuredTypeRef ref);
Upsert upsert(CqnStructuredTypeRef ref);
Update> update(CqnStructuredTypeRef ref);
Delete> delete(CqnStructuredTypeRef ref);
Select> select(CdsEntity entity);
Select> select(CdsEntity entity, UnaryOperator> path);
Insert insert(CdsEntity entity, UnaryOperator> path);
Upsert upsert(CdsEntity entity, UnaryOperator> path);
Update> update(CdsEntity entity, UnaryOperator> path);
Delete> delete(CdsEntity entity, UnaryOperator> path);
> Select select(Class entity);
, R extends StructuredType> Select select(Class entity, Function path);
> Select select(Source source);
> Insert insert(E entity);
> Upsert upsert(E entity);
> Update update(E entity);
> Delete delete(E entity);
, R extends StructuredType> Insert insert(Class entity, Function path);
, R extends StructuredType> Delete delete(Class entity, Function path);
, R extends StructuredType> Upsert upsert(Class entity, Function path);
, R extends StructuredType> Update update(Class entity, Function path);
RefBuilder copy(CqnStructuredTypeRef ref);
RefBuilder> copy(CqnElementRef ref);
Expand> copy(CqnExpand expand);
Predicate copy(CqnPredicate pred);
Predicate copy(CqnPredicate pred, Modifier modifier);
R copy(S statement);
R copy(S statement, Modifier modifier);
CqnParser parse();
QueryBuilderSupport support();
Predicate matching(Map keyValueMap);
interface QueryBuilderSupport extends Then {
Value toLower(Value val);
Value toUpper(Value val);
Parameter param(String name);
Value> plain(String val);
FunctionCall func(String functionName, Iterable extends CqnValue> args);
BooleanFunction booleanFunc(String functionName, List extends CqnValue> args);
CqnListValue list(List extends CqnValue> values);
Predicate not(CqnPredicate p);
Predicate connect(Operator operator, Iterable extends CqnPredicate> predicates);
Collector withOr();
Collector withAnd();
StructuredType> entity(String qualifiedName);
> T entity(Class type);
StructuredType> to(String path);
StructuredType> to(List extends Segment> segments);
ElementRef get(String path);
ElementRef get(List extends Segment> segments);
Segment refSegment(String id);
Segment refSegment(String id, CqnPredicate filter);
List refSegments(List segmentIds);
Value expression(CqnValue left, CqnArithmeticExpression.Operator op, CqnValue right);
Predicate comparison(CqnValue lhs, CqnComparisonPredicate.Operator op, CqnValue rhs);
Predicate in(CqnValue lhs, Collection extends CqnValue> values);
Predicate in(CqnValue lhs, CqnValue valueSet);
Predicate in(List refs, Collection extends Map> valueMaps);
Predicate in(CqnValue value, CqnSelect subquery);
Predicate between(CqnValue value, CqnValue low, CqnValue high);
Predicate search(String term);
Predicate exists(CqnSelect subQuery);
Predicate match(CqnStructuredTypeRef ref, CqnPredicate pred, Quantifier quantifier);
Value now();
Value validFrom();
Value validTo();
Value userLocale();
Value userId();
Value countDistinct(CqnValue value);
Value cosineSimilarity(CqnValue vector1, CqnValue vector2);
Value l2Distance(CqnValue vector1, CqnValue vector2);
Predicate containment(CqnContainmentTest.Position position, CqnValue value, CqnValue term,
boolean caseInsensitive);
Literal constant(T value);
Literal val(T value);
CqnVector vector(Object vector);
CqnSortSpecification sort(CqnValue value, Order order);
BooleanValue booleanValue(boolean bool);
NullValue nullValue();
Predicate matchesPattern(CqnValue value, CqnValue pattern);
Predicate matchesPattern(CqnValue value, CqnValue pattern, CqnValue options);
Predicate matchesPattern(CqnValue value, CqnValue pattern, boolean caseInsensitive, boolean multilineSensitive);
Predicate eTag(CqnListValue values);
Predicate eTag(CqnValue ref, Object value);
@Override
When when(CqnPredicate when);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy