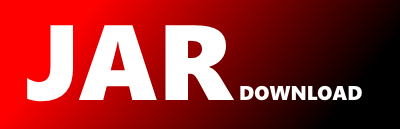
com.sap.cds.ql.Delete Maven / Gradle / Ivy
/**************************************************************************
* (C) 2019-2023 SAP SE or an SAP affiliate company. All rights reserved. *
**************************************************************************/
package com.sap.cds.ql;
import java.util.Collection;
import java.util.Map;
import java.util.function.Function;
import java.util.function.UnaryOperator;
import com.sap.cds.CdsDataStore;
import com.sap.cds.ql.cqn.CqnDelete;
import com.sap.cds.ql.cqn.CqnParameter;
import com.sap.cds.ql.cqn.CqnPredicate;
import com.sap.cds.ql.cqn.CqnStructuredTypeRef;
import com.sap.cds.reflect.CdsEntity;
/**
* The Delete Builder allows to construct CDS QL delete statements, which can be
* executed via the {@link CdsDataStore}.
*
* Note that some methods in this interface mutually exclude each other and
* cannot be combined. For example, the methods {@link #byId(Object) byId},
* {@link #byParams(String...) byParams}, {@link #matching(Map) matching}, and
* {@link #where(CqnPredicate) where} all set the where condition of the
* enclosing statement, overwriting any previously stored conditions.
*
* @param the type of the entity set targeted by this delete statement
*/
public interface Delete> extends CqnDelete, FilterableStatement> {
/**
* Creates a delete statement to delete entries from a specified entity set.
*
* @param qualifiedName the fully qualified name of the CDS entity set
* @return the delete statement
*/
static Delete> from(String qualifiedName) {
return from(qualifiedName, e -> e);
}
/**
* Creates a delete statement to delete entries from a specified entity set.
*
* @param ref the ref to the entity
* @return the delete statement
*/
static Delete> from(CqnStructuredTypeRef ref) {
return CDS.QL.builder.delete(ref);
}
/**
* Creates a delete statement to delete entries from a specified entity set.
*
* @param the type of the entity set
* @param entity the structured type representing the entity set
* @return the delete statement
*/
public static > Delete from(E entity) {
return CDS.QL.builder.delete(entity);
}
/**
* Creates a delete statement to delete entries from a specified entity set.
*
* @param rootEntityName the fully qualified name of the CDS entity set
* @param path a path expression navigating from the root entity to
* the target entity of the delete statement
* @return the delete statement
*/
static Delete> from(String rootEntityName, UnaryOperator> path) {
return CDS.QL.builder.delete(rootEntityName, path);
}
/**
* Creates a delete statement to delete entries from a specified entity set.
*
* @param entity the static model representation of the entity set
* @param the type of root entity
* @return the delete statement
*/
static > Delete from(Class entity) {
return from(entity, e -> e);
}
/**
* Creates a delete statement to delete entries from a specified entity set.
*
* @param entity the static model representation of the entity set
* @param the type of root entity
* @param the type of entity to be returned from path expression
* @param path a path expression navigating from the root entity to the target
* entity of the delete statement
* @return the delete statement
*/
static , R extends StructuredType> Delete from(Class entity,
Function path) {
return CDS.QL.builder.delete(entity, path);
}
/**
* Creates a delete statement to delete entries from a specified entity set.
*
* @param entity the model representation of the entity set obtained by
* reflection
* @return the delete statement
* @see com.sap.cds.reflect.CdsModel#findEntity(String)
* @see com.sap.cds.reflect.CdsModel#entities()
*/
static Delete> from(CdsEntity entity) {
return from(entity, e -> e);
}
/**
* Creates a delete statement to delete entries from a specified entity set.
*
* @param entity the model representation of the entity set obtained by
* reflection
* @param path a path expression navigating from the root entity to the target
* entity of the delete statement
*
* @return the delete statement
* @see com.sap.cds.reflect.CdsModel#findEntity(String)
* @see com.sap.cds.reflect.CdsModel#entities()
*/
static Delete> from(CdsEntity entity, UnaryOperator> path) {
return CDS.QL.builder.delete(entity, path);
}
/**
* Copies the given {@link CqnDelete} into a {@link Delete} builder.
*
* @param delete the {@code CqnDelete} to be copied
* @return the modifiable delete statement copy
*/
static Delete> copy(CqnDelete delete) {
return CDS.QL.builder.copy(delete);
}
/**
* Creates a delete statement to delete entries from a specified entity set.
*
* @param cqnDelete the CQN representation of delete statement
* @return the delete statement
*/
static Delete> cqn(String cqnDelete) {
return CDS.QL.parser.delete(cqnDelete);
}
/**
* Sets the where condition of this delete statement. If this delete statement
* already has a where condition it is replaced with the given.
*
* @param predicate a {@link CqnPredicate}
* @return the delete statement
*/
@Override
Delete where(CqnPredicate predicate);
/**
* Sets the where condition of this delete statement. The where condition is
* provided as a function that accepts a model object representing the entity
* targeted by the delete and returning the where condition.
*
* @param predicate a {@link Function} providing the where condition
* @return the delete statement
*/
@Override
Delete where(Function predicate);
/**
* Sets the where condition of the delete statement. The where condition is
* computed from a map of element names of the target entity set to their
* values, or a {@link CqnParameter}. The map entries are transformed into
* comparison predicates and joined via and.
*
* @param values the element name to value map defining the where condition
* @return the delete statement
*/
@Override
Delete matching(Map values);
/**
* Sets the where condition of the delete statement. The where condition is
* computed from the given vararg of element references of the target entity.
* For each element reference a predicate is created comparing the element ref
* with a {@link CqnParameter} that has the name of the ref. The predicates are
* joined via and.
*
* @param elementRefs the element references defining the where condition
* @return this delete statement
*/
@Override
Delete byParams(String... elementRefs);
/**
* Sets the where condition of the delete statement. The where condition is
* computed from the given collection of element references of the target
* entity. For each element ref a predicate is created comparing the ref with a
* {@link CqnParameter} that has the name of the ref. The predicates are joined
* via and.
*
* @param elementRefs the element references defining the where condition
* @return this delete statement
*/
@Override
Delete byParams(Collection elementRefs);
/**
* Sets the where condition of the delete statement, requiring that the value of
* the single key element of the target entity set is equal to the given
* idValue.
*
* @param idValue the value of the target entity's key element
* @return the delete statement
*/
@Override
Delete byId(Object idValue);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy