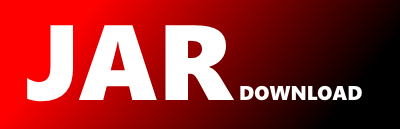
com.sap.cds.ql.RefBuilder Maven / Gradle / Ivy
/*******************************************************************
* © 2023 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.ql;
import java.util.List;
import java.util.stream.Stream;
import com.sap.cds.ql.cqn.CqnPredicate;
import com.sap.cds.ql.cqn.CqnReference;
/**
* Builder for CQN {@link CqnReference references}.
*
* The {@link RefSegment segments} of the ref can be modified in-place. Call
* {@link #build} to create an immutable ref.
*/
public interface RefBuilder {
/**
* Returns the segments of this ref builder as {@link List}.
*
* @return the list of {@link RefSegment RefSegments}
*/
List segments();
/**
* Returns the segments of this ref builder as {@link Stream}.
*
* @return the stream of {@link RefSegment RefSegments}
*/
Stream stream();
/**
* Returns the first segment of this ref builder.
*
* @return the first {@link RefSegment}
*/
RefSegment rootSegment();
/**
* Returns the last segment of this ref builder.
*
* @return the last {@link RefSegment}
*/
RefSegment targetSegment();
/**
* Sets the alias of this ref builder.
*
* @param alias the alias
* @return this ref builder
*/
RefBuilder as(String alias);
/**
* Sets the type of this ref builder.
*
* @param typeName the type name
* @return this ref builder
*/
RefBuilder type(String typeName);
/**
* Creates an immutable ref
*
* @return an immutable ref of type T
*/
T build();
/**
* A modifiable ref segment.
*/
interface RefSegment extends CqnReference.Segment {
/**
* Sets the id of this segment.
*
* @param id the new segment id
* @return this ref segment
*/
RefSegment id(String id);
/**
* Sets the filter of this segment.
*
* @param filter the new segment filter
* @return this ref segment
*/
RefSegment filter(CqnPredicate filter);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy