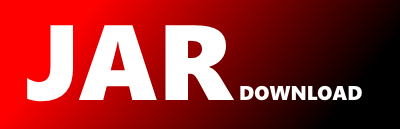
com.sap.cds.ql.StatementBuilder Maven / Gradle / Ivy
/*******************************************************************
* © 2022 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.ql;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import com.sap.cds.ql.cqn.CqnStatement;
public abstract class StatementBuilder implements CqnStatement {
protected final Map hints = new HashMap<>(0);
@Override
public Map hints() {
return Collections.unmodifiableMap(hints);
}
/**
* Sets the hints for the statement.
*
* @param hints the hint map
* @return this statement
*/
@SuppressWarnings("unchecked")
public T hints(Map hints) {
this.hints.clear();
this.hints.putAll(hints);
return (T) this;
}
/**
* Adds hints to the statement.
*
* @param hints the hints
* @return this statement
*/
@SuppressWarnings("unchecked")
public T hints(String... hints) {
for (String hint : hints) {
hint(hint, true);
}
return (T) this;
}
/**
* Adds a hint to the statement.
*
* @param name the hint name
* @param value the hint value
* @return this statement
*/
@SuppressWarnings("unchecked")
public T hint(String name, Object value) {
this.hints.put(name, value);
return (T) this;
}
@Override
public String toString() {
return toJson();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy