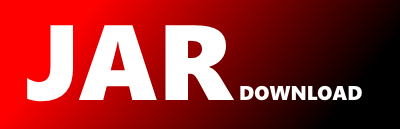
com.sap.cds.ql.Upsert Maven / Gradle / Ivy
/************************************************************************
* © 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
************************************************************************/
package com.sap.cds.ql;
import java.util.Map;
import java.util.function.Function;
import java.util.function.UnaryOperator;
import com.sap.cds.CdsDataStore;
import com.sap.cds.ql.cqn.CqnStructuredTypeRef;
import com.sap.cds.ql.cqn.CqnUpsert;
import com.sap.cds.reflect.CdsEntity;
import com.sap.cds.reflect.CdsModel;
/**
* The Upsert builder allows to construct a CDS QL Upsert statements, which can
* be executed via the {@link CdsDataStore}.
*
* An Upsert statement updates an entity from the given data. The key of the entity
* that is upserted is expected to be in the data. If the entity doesn't exist and the key
* is given in the data, it inserts a new entry.
*/
public interface Upsert extends CqnUpsert, Statement {
/**
* Creates an upsert statement to upsert entries into a specified entity set.
*
* @param qualifiedName the fully qualified name of the CDS entity set
* @return the upsert statement
*/
static Upsert into(String qualifiedName) {
return into(qualifiedName, e -> e);
}
/**
* Creates an upsert statement to upsert entries into a specified entity set.
*
* @param ref the ref to the entity
* @return the upsert statement
*/
static Upsert into(CqnStructuredTypeRef ref) {
return CDS.QL.builder.upsert(ref);
}
/**
* Creates an upsert statement to upsert entries into a specified entity set.
*
* @param the type of the entity set
* @param entity the structured type representing the entity set
* @return the upsert statement
*/
public static > Upsert into(T entity) {
return CDS.QL.builder.upsert(entity);
}
/**
* Creates an upsert statement to upsert entries into a specified entity set.
*
* @param qualifiedName the fully qualified name of the CDS entity set
* @param path a path expression navigating from the root entity to the
* target entity of the upsert statement
* @return the upsert statement
*/
static Upsert into(String qualifiedName, UnaryOperator> path) {
return CDS.QL.builder.upsert(qualifiedName, path);
}
/**
* Creates an upsert statement to upsert entries into a specified entity set.
*
* @param the type of the entity set
* @param entity the static model representation of the entity set
* @return the upsert statement
*/
static > Upsert into(Class entity) {
return into(entity, e -> e);
}
/**
* Creates an upsert statement to upsert entries into a specified entity set.
*
* @param the type of the root entity
* @param the type of the target entity
* @param entity the static model representation of the entity set
* @param path a path expression navigating from the root entity to the target
* entity of the upsert statement
* @return the upsert statement
*/
static , T extends StructuredType> Upsert into(Class entity,
Function path) {
return CDS.QL.builder.upsert(entity, path);
}
/**
* Creates an upsert statement to upsert entries into a specified entity set.
*
* @param entity the model representation of the entity set obtained by
* reflection
* @return the upsert statement
* @see com.sap.cds.reflect.CdsModel#findEntity(String)
* @see com.sap.cds.reflect.CdsModel#entities()
*/
static Upsert into(CdsEntity entity) {
return into(entity, e -> e);
}
/**
* Creates an upsert statement to upsert entries into a specified entity set.
*
* @param entity the model representation of the entity set obtained by
* reflection
* @param path a path expression navigating from the root entity to the target
* entity of the upsert statement
* @return the upsert statement
* @see com.sap.cds.reflect.CdsModel#findEntity(String)
* @see com.sap.cds.reflect.CdsModel#entities()
*/
static Upsert into(CdsEntity entity, UnaryOperator> path) {
return CDS.QL.builder.upsert(entity, path);
}
/**
* Copies the given {@link CqnUpsert} into an {@link Upsert} builder.
*
* @param upsert the {@code CqnUpsert} to be copied
* @return the modifiable upsert statement copy
*/
static Upsert copy(CqnUpsert upsert) {
return CDS.QL.builder.copy(upsert);
}
/**
* Creates an upsert statement to upsert data for a specified entity set.
*
* @param model the CDS model
* @param cqnUpsert the CQN representation of the upsert statement
* @return the upsert statement
*/
static Upsert cqn(CdsModel model, String cqnUpsert) {
return CDS.QL.parser.upsert(model, cqnUpsert);
}
/**
* Sets the batch data to be inserted or updated by the upsert statement. The
* data is given as a collection of maps from element names of the target entity
* set to their new values. The data must contain the entities' keys.
*
* @param entries a collection of data where every element is the canonical
* representation
* @return the upsert statement
*/
Upsert entries(Iterable extends Map> entries);
/**
* Adds a single entry to be upserted into the entity set. The data is given as
* a {@literal Map} that maps element names of the target entity
* set to the values to be upserted.
*
* The value can be deeply structured to represent a structured document:
*
* - for single-valued relationships the value of the element is of type
* {@literal Map
}
* - for collection-valued relationships the value of the element is of type
* {@literal List
*
*
* @param entry the data to be upserted as a map
*
* @return the upsert statement
*/
Upsert entry(Map entry);
/**
* Adds a single entry to be upserted into the entity set.
*
* The value can be deeply structured to represent a structured document:
*
* - for single-valued relationships the value of the element is of type
* {@literal Map
}
* - for collection-valued relationships the value of the element is of type
* {@literal List
*
*
* @param elementName the element name of the target entity for the value to be
* upserted
* @param value the data to be upserted for the element
*
* @return the upsert statement
*/
Upsert entry(String elementName, Object value);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy