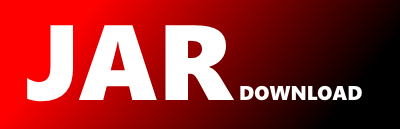
com.sap.cds.ql.Value Maven / Gradle / Ivy
/************************************************************************
* © 2019-2023 SAP SE or an SAP affiliate company. All rights reserved. *
************************************************************************/
package com.sap.cds.ql;
import java.util.List;
import com.sap.cds.ql.cqn.CqnSelect;
import com.sap.cds.ql.cqn.CqnSelectListValue;
import com.sap.cds.ql.cqn.CqnValue;
import com.sap.cds.reflect.CdsBaseType;
public interface Value extends CqnValue, Orderable {
/**
* Creates an expression that adds a given summand to this numeric value
*
* @param s the summand
* @return expression for the addition
*/
Value plus(Number s);
/**
* Creates an expression that adds a given summand to this numeric value
*
* @param s the summand
* @return expression for the addition
*/
Value plus(Value extends Number> s);
/**
* Creates an expression that subtracts a given subtrahend from this numeric
* value
*
* @param s the subtrahend
* @return expression for the subtraction
*/
Value minus(Number s);
/**
* Creates an expression that subtracts a given subtrahend from this numeric
* value
*
* @param s the subtrahend
* @return expression for the subtraction
*/
Value minus(Value extends Number> s);
/**
* Creates an expression that multiplies this numeric value with a given factor
*
* @param f the factor
* @return expression for the multiplication
*/
Value times(Number f);
/**
* Creates an expression that multiplies this numeric value with a given factor
*
* @param f the factor
* @return expression for the multiplication
*/
Value times(Value extends Number> f);
/**
* Creates an expression that divides this numeric value by a given divisor
*
* @param d the divisor
* @return expression for the division
*/
Value dividedBy(Number d);
/**
* Creates an expression that divides this numeric value by a given divisor
*
* @param d the divisor
* @return expression for the division
*/
Value dividedBy(Value extends Number> d);
/**
* Creates an expression for substring extraction from this value. Extracts a
* substring of given length starting at a specified position. First position is
* 0.
*
* @param start the start position
* @param length the number of characters that you want to extract
* @return expression for substring extraction
*/
Value substring(Value start, Value length);
/**
* Creates an expression for substring extraction from this string value.
* Extracts a substring of given length starting at a specified position. First
* position is 0.
*
* @param start the start position
* @param length the number of characters that you want to extract
* @return expression for substring extraction
*/
Value substring(int start, int length);
/**
* Creates an expression for substring extraction from this string value.
* Extracts a substring starting at a specified position extending to the end of
* the string. First position is 0.
*
* @param start the start position
* @return the expression for substring extraction
*/
Value substring(Value start);
/**
* Creates an expression for substring extraction from this string value.
* Extracts a substring starting at a specified position extending to the end of
* the string. First position is 0.
*
* @param start the start position
* @return the expression for substring extraction
*/
Value substring(int start);
/**
* Creates an expression for converting this string value to upper case using
* the rules of the underlying data store.
*
* @return the String value in UpperCase
*/
Value toUpper();
/**
* Creates an expression for converting this string value to lower case using
* the rules of the underlying data store.
*
* @return the String value in LowerCase
*/
Value toLower();
/**
* Creates a function by passing this value as a parameter to the underlying
* data store's MIN function.
*
* @return the minimum value
*/
Value min();
/**
* Creates a function by passing this value as a parameter to the underlying
* data store's MAX function.
*
* @return the maximum value
*/
Value max();
/**
* Creates a function by passing this value as a parameter to the underlying
* data store's SUM function.
*
* @return the summation number value
*/
Value sum();
/**
* Creates a function by passing this value as a parameter to the underlying
* data store's AVG function.
*
* @return the average number value
*/
Value average();
/**
* Creates a function by passing this value as a parameter with the DISTINCT
* keyword to the underlying data store's COUNT function.
*
* @return the countDistinct long value
*/
Value countDistinct();
/**
* Creates a predicate that tests if this value is NULL
*
* @return the predicate for the NULL test
*/
Predicate isNull();
/**
* Creates a predicate that tests if this value is not NULL
*
* @return the predicate for the not NULL test
*/
Predicate isNotNull();
/**
* Creates a comparison predicate that tests if this value is equal to a given
* value. Depending on the underlying datastore, NULL values might be
* treated as unknown resulting in a three-valued logic as in SQL.
*
* @param v the value to compare with
* @return the comparison predicate
*/
Predicate eq(T v);
/**
* Creates a comparison predicate that tests if this value is equal to a given
* value. Depending on the underlying datastore, NULL values might be
* treated as unknown resulting in a three-valued logic as in SQL.
*
* @param v the value to compare with
* @return the comparison predicate
*/
Predicate eq(Value v);
/**
* Creates a comparison predicate that tests if this value is not equal to a
* given value. Depending on the underlying datastore, NULL values might be
* treated as unknown resulting in a three-valued logic as in SQL.
*
* @param v the value to compare with
* @return the comparison predicate
*/
Predicate ne(T v);
/**
* Creates a comparison predicate that tests if this value is not equal to a
* given value. Depending on the underlying datastore, NULL values might be
* treated as unknown resulting in a three-valued logic as in SQL.
*
* @param v the value to compare with
* @return the comparison predicate
*/
Predicate ne(Value v);
/**
* Creates a comparison predicate that tests if this value is equal to a given
* value. NULL values are treated as any other value.
*
* @param v the value to compare with
* @return the comparison predicate
*/
Predicate is(T v);
/**
* Creates a comparison predicate that tests if this value is equal to a given
* value. NULL values are treated as any other value.
*
* @param v the value to compare with
* @return the comparison predicate
*/
Predicate is(Value v);
/**
* Creates a comparison predicate that tests if this value is not equal to a
* given value. NULL values are treated as any other value.
*
* @param v the value to compare with
* @return the comparison predicate
*/
Predicate isNot(T v);
/**
* Creates a comparison predicate that tests if this value is not equal to a
* given value. NULL values are treated as any other value.
*
* @param v the value to compare with
* @return the comparison predicate
*/
Predicate isNot(Value v);
/**
* Creates a comparison predicate that tests if this value is greater than a
* given value
*
* @param v the value to compare with
* @return the comparison predicate
*/
Predicate gt(T v);
/**
* Creates a comparison predicate that tests if this value is greater than a
* given value
*
* @param v the value to compare with
* @return the comparison predicate
*/
Predicate gt(Value v);
/**
* Creates a comparison predicate that tests if this value is greater than or
* equal to a given value
*
* @param v the value to compare with
* @return the comparison predicate
*/
Predicate ge(T v);
/**
* Creates a comparison predicate that tests if this value is greater than or
* equal to a given value
*
* @param v the value to compare with
* @return the comparison predicate
*/
Predicate ge(Value v);
/**
* Creates a comparison predicate that tests if this value is less than a given
* value
*
* @param v the value to compare with
* @return the comparison predicate
*/
Predicate lt(T v);
/**
* Creates a comparison predicate that tests if this value is less than a given
* value
*
* @param v the value to compare with
* @return the comparison predicate
*/
Predicate lt(Value v);
/**
* Creates a comparison predicate that tests if this value is less than or equal
* to a given value
*
* @param v the value to compare with
* @return the comparison predicate
*/
Predicate le(T v);
/**
* Creates a comparison predicate that tests if this value is less than or equal
* to a given value
*
* @param v the value to compare with
* @return the comparison predicate
*/
Predicate le(Value v);
/**
* Creates a between predicate that tests if this value is within a closed
* interval with given bounds
*
* @param low the lower bound
* @param high the upper bound
* @return the between predicate
*/
Predicate between(T low, T high);
/**
* Creates a between predicate that tests if this value is within a closed
* interval with given bounds
*
* @param low the lower bound
* @param high the upper bound
* @return the between predicate
*/
Predicate between(Value low, T high);
/**
* Creates a between predicate that tests if this value is within a closed
* interval with given bounds
*
* @param low the lower bound
* @param high the upper bound
* @return the between predicate
*/
Predicate between(T low, Value high);
/**
* Creates a between predicate that tests if this value is within a closed
* interval with given bounds
*
* @param low the lower bound
* @param high the upper bound
* @return the between predicate
*/
Predicate between(Value low, Value high);
/**
* Creates an IN predicate that tests if this value is equal to any value in a
* given list
*
* @param vs the given values
* @return the IN predicate for the in list test
*/
Predicate in(@SuppressWarnings("unchecked") T... vs);
/**
* Creates an IN predicate that tests if this value is equal to any value in a
* given list
*
* @param ts the given values
* @return the IN predicate for the in list test
*/
Predicate in(List extends T> ts);
/**
* Creates an IN predicate that tests if this value is equal to any value in a
* given list
*
* @param ts the given values
* @return the IN predicate for the in list test
*/
Predicate in(@SuppressWarnings("unchecked") Value... ts);
/**
* Creates an IN predicate that tests if this value is equal to any value in a
* given list
*
* @param ts the given values
* @return the IN predicate for the in list test
*/
Predicate in(Iterable> ts);
/**
* Creates an IN predicate that tests if this value is equal to any value
* returned by the subquery.
*
* @param subquery the subquery that defines the set of expected values
* @return the IN predicate
*/
Predicate in(CqnSelect subquery);
Predicate plain(Object... p);
/**
* Creates a predicate that applies a given function to this values and optional
* additional values
*
* @param functionName the function name
* @param additional the additional values
* @return the function call
*/
Predicate func(String functionName, Value>... additional);
/**
* Creates a predicate that tests whether this string value contains a given
* substring
*
* @param substring the substring
* @return the predicate for the containment test
*/
Predicate contains(String substring);
/**
* Creates a predicate that tests whether this string value contains a given
* substring
*
* @param substring the substring
* @return the predicate for the containment test
*/
Predicate contains(Value substring);
/**
* Creates a predicate that tests whether this string value contains a given
* substring
*
* @param substring the substring
* @param caseInsensitive whether the test should be case insensitive
* @return the predicate for the containment test
*/
Predicate contains(Value substring, boolean caseInsensitive);
/**
* Creates a predicate that tests whether this string value starts with a given
* prefix
*
* @param prefix the prefix
* @return the predicate for the starts-with test
*/
Predicate startsWith(String prefix);
/**
* Creates a predicate that tests whether this string value starts with a given
* prefix
*
* @param prefix the prefix
* @return the predicate for the starts-with test
*/
Predicate startsWith(Value prefix);
/**
* Creates a predicate that tests whether this string value ends with a given
* suffix
*
* @param suffix the suffix
* @return the predicate for the ends-with test
*/
Predicate endsWith(String suffix);
/**
* Creates a predicate that tests whether this string value ends with a given
* suffix
*
* @param suffix the suffix
* @return the predicate for the ends-with test
*/
Predicate endsWith(Value suffix);
CqnSelectListValue as(String alias);
CqnSelectListValue withoutAlias();
/**
* Sets the type.
*
* @param javaType the Java type
* @return a value with the given type. Can be a new value if this value is
* immutable
*/
Value type(Class javaType);
/**
* Sets the type.
*
* @param cdsType the CDS type
* @return a value with the given type. Can be a new value if this value is
* immutable
*/
Value type(CdsBaseType cdsType);
/**
* Sets the type.
*
* @param cdsType the CDS type name
* @return a value with the given type. Can be a new value if this value is
* immutable
*/
Value type(String cdsType);
/**
* Creates a predicate that tests if this value matches a specified regular expression
*
* @param pattern regular expression represented as a string value
* @return the predicate to match the expression
*/
Predicate matchesPattern(Value pattern);
/**
* Creates a predicate that tests if this value matches a specified regular expression
*
* @param pattern regular expression represented as a plain string
* @return the predicate to match the expression
*/
Predicate matchesPattern(String pattern);
/**
* Creates a predicate that tests if this value matches a specified regular expression
*
* @param pattern regular expression represented as a string value
* @param options regular expression options represented as a string value
* @return the predicate to match the expression
*/
Predicate matchesPattern(Value pattern, Value options);
/**
* Creates a predicate that tests if this value matches a specified regular expression
*
* @param pattern regular expression represented as a string value
* @param caseInsensitive whether the test is case-insensitive
* @param multiLineSensitive whether the test is multi-line sensitive
* @return the predicate to match the expression
*/
Predicate matchesPattern(Value pattern, boolean caseInsensitive, boolean multiLineSensitive);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy