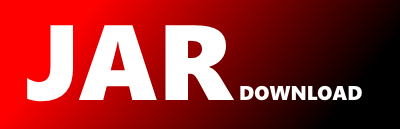
com.sap.cds.ql.cqn.CqnAnalyzer Maven / Gradle / Ivy
/*******************************************************************
* © 2020 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.ql.cqn;
import java.util.function.Supplier;
import com.google.common.annotations.Beta;
import com.sap.cds.Cds4jServiceLoader;
import com.sap.cds.ql.cqn.CqnReference.Segment;
import com.sap.cds.reflect.CdsModel;
/**
* API to introspect {@link CqnStructuredTypeRef CqnStructuredTypeRef(s)} and
* {@link CqnStatement CqnStatement(s)}
*/
public final class CqnAnalyzer {
private static final CqnAnalyzerSPI analyzer = Cds4jServiceLoader.load(CqnAnalyzerSPI.class);
private final Supplier modelSupplier;
private CqnAnalyzer(Supplier modelSupplier) {
this.modelSupplier = modelSupplier;
}
/**
* Returns true if the given CQN statement is a query with a single count item
* on the select list.
*
* @param cqn the CQN statement
* @return true if the given statement is a count query
*/
public static boolean isCountQuery(CqnStatement cqn) {
return analyzer.isCountQuery(cqn);
}
/**
* Creates an instance of {@code CqnAnalyzer}.
*
* @param model the {@link CdsModel}
* @return the {@code CqnAnalyzer} instance
*/
public static CqnAnalyzer create(CdsModel model) {
return create(() -> model);
}
/**
* Creates an instance of {@code CqnAnalyzer}.
*
* @param modelSupplier the {@link CdsModel} supplier
* @return the {@code CqnAnalyzer} instance
*/
public static CqnAnalyzer create(Supplier modelSupplier) {
return new CqnAnalyzer(modelSupplier);
}
/**
* Analyzes the {@link Segment Segment(s)} of the given
* {@link CqnStructuredTypeRef}.
*
* @param ref the {@code CqnStructuredTypeRef} to be analyzed
* @return the {@link AnalysisResult} with information on the segments of the
* {@code CqnStructuredTypeRef}
*/
public AnalysisResult analyze(CqnStructuredTypeRef ref) {
return analyzer.analyze(modelSupplier.get(), ref);
}
/**
* Analyzes the {@code ref} and {@code where} condition of the given
* {@link CqnFilterableStatement}.
*
* @param statement the {@link CqnFilterableStatement} statement to be analyzed
* ({@link CqnDelete}, {@link CqnUpdate}, or {@link CqnSelect})
* @return the {@link AnalysisResult} with information on the {@code ref}
* {@link Segment Segment(s)} and {@code where} condition of the filterable statement
*/
public AnalysisResult analyze(CqnFilterableStatement statement) {
return analyzer.analyze(modelSupplier.get(), statement);
}
@Beta
public Iterable resolveRefItems(CqnSelect query) {
return analyzer.resolveRefItems(modelSupplier.get(), query);
}
/**
* Service Provider Interface for the {@link CqnAnalyzer}.
*/
public interface CqnAnalyzerSPI {
AnalysisResult analyze(CdsModel model, CqnStructuredTypeRef ref);
AnalysisResult analyze(CdsModel model, CqnFilterableStatement stmt);
Iterable resolveRefItems(CdsModel model, CqnSelect query);
boolean isCountQuery(CqnStatement cqn);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy