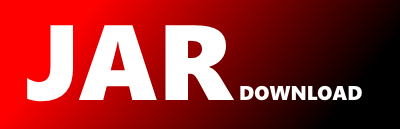
com.sap.cds.ql.cqn.CqnLiteral Maven / Gradle / Ivy
/************************************************************************
* © 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
************************************************************************/
package com.sap.cds.ql.cqn;
import java.util.stream.Stream;
import com.google.common.annotations.Beta;
/**
* A CQN literal value of type {@link T}. Use {@link #value} to get the value.
*/
public interface CqnLiteral extends CqnValue {
/**
* Returns the literal value.
*
* @return the literal value
*/
T value();
/**
* Specifies if this {@link CqnLiteral} should be handled as a constant value
* during query execution.
*
* @return true if the literal can be handled as a constant value
*/
default boolean isConstant() {
return true;
}
@Override
default CqnLiteral> asLiteral() {
return this;
}
@Override
default boolean isLiteral() {
return true;
}
@Override
default Stream> ofLiteral() {
return Stream.of(this);
}
/**
* Returns {@code true} if this is a {@link CqnBooleanLiteral}.
*
* @return {@code true} if this is a boolean literal, otherwise {@code false}
*/
default boolean isBoolean() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnNumericLiteral}.
*
* @return {@code true} if this is a numeric literal, otherwise {@code false}
*/
default boolean isNumeric() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnStringLiteral}.
*
* @return {@code true} if this is a string literal, otherwise {@code false}
*/
default boolean isString() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnTemporalLiteral}.
*
* @return {@code true} if this is a temporal literal, otherwise {@code false}
*/
default boolean isTemporal() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnStructuredLiteral}.
*
* @return {@code true} if this is a structured literal, otherwise {@code false}
*/
default boolean isStructured() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnVector}.
*
* @return {@code true} if this is a vector, otherwise {@code false}
*/
@Beta
default boolean isVector() {
return false;
}
/**
* Casts this literal to {@code CqnBooleanLiteral}.
*
* @throws ClassCastException if this literal is not boolean
* @return this literal as a boolean literal
*/
default CqnBooleanLiteral asBoolean() {
return as(CqnBooleanLiteral.class);
}
/**
* Casts this literal to {@code CqnStringLiteral}.
*
* @throws ClassCastException if this literal is not a string literal
* @return this literal as a string literal
*/
default CqnStringLiteral asString() {
return as(CqnStringLiteral.class);
}
/**
* Casts this literal to {@code CqnNumericLiteral}.
*
* @throws ClassCastException if this literal is not numeric
* @return this literal as a numeric literal
*/
default CqnNumericLiteral> asNumber() {
return as(CqnNumericLiteral.class);
}
/**
* Casts this literal to {@code CqnTemporalLiteral}.
*
* @throws ClassCastException if this literal is not temporal
* @return this literal as a temporal literal
*/
default CqnTemporalLiteral> asTemporal() {
return as(CqnTemporalLiteral.class);
}
/**
* Casts this literal to {@code CqnStructuredLiteral}.
*
* @throws ClassCastException if this literal is not structured
* @return this literal as a structured literal
*/
default CqnStructuredLiteral asStructured() {
return as(CqnStructuredLiteral.class);
}
/**
* Casts this literal to {@code CqnVector}.
*
* @throws ClassCastException if this literal is no vector
* @return this literal as a vector
*/
default CqnVector asVector() {
return as(CqnVector.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy