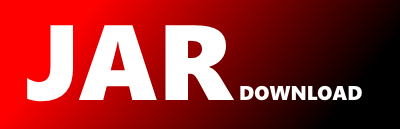
com.sap.cds.ql.cqn.CqnValue Maven / Gradle / Ivy
/*******************************************************************
* © 2019 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.ql.cqn;
import java.util.Optional;
import java.util.stream.Stream;
public interface CqnValue extends CqnToken {
/**
* Returns the optional CDS type of this value.
*
* @return the optional CDS type
*/
default Optional type() {
return Optional.empty();
}
/**
* Returns {@code true} if this is a {@link CqnElementRef}.
*
* @return {@code true} if this is an element ref, otherwise {@code false}
*/
default boolean isRef() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnExpression}.
*
* @return {@code true} if this is an expression, otherwise {@code false}
*/
default boolean isExpression() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnFunc}.
*
* @return {@code true} if this is a function, otherwise {@code false}
*/
default boolean isFunction() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnLiteral}.
*
* @return {@code true} if this is a literal, otherwise {@code false}
*/
default boolean isLiteral() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnNullValue}.
*
* @return {@code true} if this is a null value, otherwise {@code false}
*/
default boolean isNullValue() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnParameter}.
*
* @return {@code true} if this is a parameter, otherwise {@code false}
*/
default boolean isParameter() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnPlain} value.
*
* @return {@code true} if this is a plain value, otherwise {@code false}
*/
default boolean isPlain() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnListValue} value.
*
* @return {@code true} if this is a list value, otherwise {@code false}
*/
default boolean isList() {
return false;
}
/**
* Casts this value to {@code CqnElementRef}.
*
* @throws ClassCastException if this value is not an element ref
* @return this value as an element ref
*/
default CqnElementRef asRef() {
throw new ClassCastException("Cannot cast to CqnElementRef");
}
default Stream ofRef() {
return Stream.empty();
}
/**
* Casts this value to {@code CqnExpression}.
*
* @throws ClassCastException if this value is not an expression
* @return this value as an expression
*/
default CqnExpression asExpression() {
throw new ClassCastException("Cannot cast to CqnExpression");
}
/**
* Casts this value to {@code CqnFunc}.
*
* @throws ClassCastException if this value is not a function
* @return this value as a function
*/
default CqnFunc asFunction() {
throw new ClassCastException("Cannot cast to CqnFunc");
}
/**
* Casts this value to {@code CqnLiteral}.
*
* @throws ClassCastException if this value is not a literal
* @return this value as a literal
*/
default CqnLiteral> asLiteral() {
throw new ClassCastException("Cannot cast to CqnLiteral");
}
default Stream> ofLiteral() {
return Stream.empty();
}
/**
* Casts this value to {@code CqnNullValue}.
*
* @throws ClassCastException if this value is not a null value
* @return this value as a null value
*/
default CqnNullValue asNullValue() {
throw new ClassCastException("Cannot cast to CqnNullValue");
}
/**
* Casts this value to {@code CqnParameter}.
*
* @throws ClassCastException if this value is not a parameter
* @return this value as a parameter
*/
default CqnParameter asParameter() {
throw new ClassCastException("Cannot cast to CqnParameter");
}
/**
* Casts this value to {@code CqnPlain}.
*
* @throws ClassCastException if this value is not a plain value
* @return this value as a plain value
*/
default CqnPlain asPlain() {
throw new ClassCastException("Cannot cast to CqnPlain");
}
/**
* Casts this value to {@code CqnListValue}.
*
* @throws ClassCastException if this value is not a list value
* @return this value as a list value
*/
default CqnListValue asList() {
throw new ClassCastException("Cannot cast to CqnListValue");
}
/**
* Casts this type to the given {@code type}.
*
* @param the return type
* @param type the subtype of {@code CqnValue} to cast to
* @return this type casted to the given {@code type} class
*/
@SuppressWarnings("unchecked")
default T as(Class type) {
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy