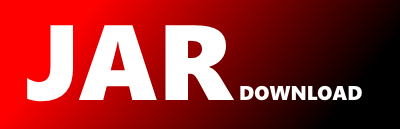
com.sap.cds.ql.hana.Hierarchy Maven / Gradle / Ivy
/*******************************************************************
* © 2024 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.ql.hana;
import java.util.List;
import com.google.common.annotations.Beta;
import com.sap.cds.ql.Source;
import com.sap.cds.ql.TableFunction;
import com.sap.cds.ql.cqn.CqnPredicate;
import com.sap.cds.ql.cqn.CqnSortSpecification;
/**
* A SAP HANA hierarchy generator function that generates a hierarchy based on recursive
* parent-child source data.
*
* The function adds the following elements to the result:
*
*
* Added elements
* element name type description
* hierarchy_rank Int64 The preorder rank of the node in the tree representation of the result set
* hierarchy_level Int32 The level to which the node belongs
* hierarchy_tree_size Int64 The number of descendant nodes +1
*
*/
@Beta
public interface Hierarchy extends CqnHierarchy, TableFunction {
/**
* Creates a copy of this hierarchy generator function with the given source.
*
* @param source the hierarchy source
* @return new hierarchy generator function with the given source
*/
Hierarchy copy(Source> source);
/**
* Specifies a filter condition that identifies the root nodes of the hierarchy
* generated by this generator. If no filter is specified the root nodes
* are identified by the condition `parent_id = NULL`.
*
* @param startWhere the filter condition
* @return this hierarchy generator function
*/
Hierarchy startWhere(CqnPredicate startWhere);
/**
* Specifies the sort order of sibling nodes. This overrides any sort order the
* source might originally have.
*
* @param siblingOrderBy the sort specifications
* @return this hierarchy generator function
*/
Hierarchy orderBy(CqnSortSpecification... siblingOrderBy);
/**
* Specifies the sort order of sibling nodes. This overrides any sort order the
* source might originally have.
*
* @param siblingOrderBy the sort specifications
* @return this hierarchy generator function
*/
Hierarchy orderBy(List siblingOrderBy);
/**
* Specifies the sort order of sibling nodes given as element names of the
* source of this hierarchy. This overrides any sort order the source might
* originally have.
*
* @param siblingOrderBy the element names
* @return this hierarchy generator function
*/
Hierarchy orderBy(String... siblingOrderBy);
/**
* Specifies the maximum search depth during hierarchy generation.
* @param depth the maximum depth
* @return this hierarchy generator function
*/
Hierarchy depth(Integer depth);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy