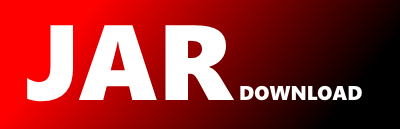
com.sap.cds.reflect.CdsDefinition Maven / Gradle / Ivy
/*******************************************************************
* © 2019 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.reflect;
import java.util.function.Predicate;
public interface CdsDefinition extends CdsAnnotatable, CdsNamed {
/**
* Accepts a {@link CdsVisitor} visiting the elements of this definition
* (depth-first)
*
* @param visitor the {@code CdsVisitor}
*/
void accept(CdsVisitor visitor);
/**
* Returns the fully qualified name of this definition.
*
* @return the fully qualified name of this definition
*/
String getQualifiedName();
/**
* Returns the unqualified name of this definition.
*
* @return the unqualified name
*/
String getName();
/**
* Returns the qualifier part of this definition's qualified name.
*
* @return the qualifier of the qualified name, or an empty string if this definition does not have a qualifier
*/
String getQualifier();
/**
* Returns the kind of this definition.
*
* @return the kind of this definition
*/
CdsKind getKind();
/**
* Returns a {@link Predicate} to filter {@code CdsDefinition(s)} that are
* inside the given namespace. If the namespace is empty or {@code null}, all
* definitions match.
*
* @param namespace the namespace to filter, can be empty or {@code null}
* @return a {@link Predicate} filtering by namespace
*/
static Predicate byNamespace(String namespace) {
if (namespace == null || namespace.isEmpty()) {
return d -> true;
}
return d -> d.getQualifiedName().startsWith(namespace + ".");
}
/**
* Casts this type to the given {@code CdsDefinition}.
*
* @param the return type
* @param type the subtype of {@code CdsDefinition} to cast to
* @return this type casted to the given {@code CdsDefinition} class
*/
@SuppressWarnings("unchecked")
default T as(Class type) {
return (T) this;
}
@Override
default String getKey() {
return getQualifiedName();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy