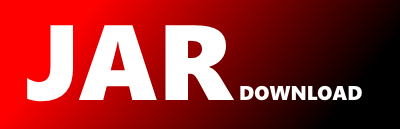
com.sap.cds.reflect.CdsElementDefinition Maven / Gradle / Ivy
/*********************************************************************
* (C) 2024 SAP SE or an SAP affiliate company. All rights reserved. *
*********************************************************************/
package com.sap.cds.reflect;
import java.util.Optional;
import com.sap.cds.ql.cqn.CqnValue;
public interface CdsElementDefinition extends CdsAnnotatable, CdsNamed {
/**
* Returns the name of this {@code CdsElement}.
*
* @return the namespace of this element, not {@code null}
*/
String getName();
/**
* Returns the type of this {@code CdsElement}.
*
* @param the {@link CdsType} of this element
* @return the type of this element, not {@code null}
*/
T getType();
/**
* Returns {@code true} if this {@code CdsElement} is a key.
*
* @return {@code true} if this element is a key, otherwise {@code false}
*/
boolean isKey();
/**
* Returns {@code true} if this {@code CdsElement} is virtual.
*
* @return {@code true} if this is a virtual element, otherwise {@code false}
*/
boolean isVirtual();
/**
* Returns {@code true} if this {@code CdsElement} is unique.
*
* @return {@code true} if this is a unique element, otherwise {@code false}
*/
boolean isUnique();
/**
* Returns {@code true} if this {@code CdsElement} cannot be {@code null}.
*
* @return {@code true} if this element cannot be {@code null}, otherwise
* {@code false}
*/
boolean isNotNull();
/**
* Returns {@code true} if this {@code CdsElement} is localized.
*
* @return {@code true} if this element is localized, otherwise {@code false}
*/
boolean isLocalized();
/**
* Returns an {@link Optional} wrapping the default value of this element.
*
* @return an {@code Optional} describing the default value of this element, or
* an empty {@code Optional} if there is no default value
*/
Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy