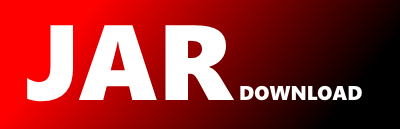
com.sap.cds.reflect.CdsEntity Maven / Gradle / Ivy
/*******************************************************************
* © 2019 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.reflect;
import java.util.Optional;
import java.util.stream.Stream;
import com.sap.cds.ql.cqn.CqnSelect;
public interface CdsEntity extends CdsStructuredType {
/**
* Returns {@code true} if this entity is abstract.
*
* @return {@code true} if this entity is abstract, otherwise {@code false}
*/
boolean isAbstract();
/**
* Returns {@code true} if this entity is a view.
*
* @return {@code true} if this entity is a view, otherwise {@code false}
*/
boolean isView();
/**
* Returns {@code true} if this entity is a projection.
*
* @return {@code true} if this entity is a projection, otherwise {@code false}
*/
boolean isProjection();
/**
* Returns the query of this {@code CdsEntity}.
*
* @return an {@code Optional} describing the query, or an empty
* {@code Optional} if there is no query
* @see CqnSelect
*/
Optional query();
/**
* Returns a sequential {@code Stream} over the {@link CdsParameter
* CdsParameter(s)} of this {@code CdsEntity}.
*
* @return a sequential {@code Stream} over the {@code CdsParameter(s)}
*/
Stream params();
/**
* Returns a sequential {@code Stream} over the bounded {@link CdsAction
* CdsAction(s)} of this {@code CdsEntity}.
*
* @return a sequential {@code Stream} over the {@code CdsAction(s)}
*/
Stream actions();
/**
* Get a bounded {@link CdsAction} via its name.
*
* @param name the name of the {@code CdsAction}
* @return the {@code CdsAction} with the given name
* @throws CdsOperationNotFoundException if there is no {@code CdsAction} with
* the given name
*/
CdsAction getAction(String name);
/**
* Returns an {@link Optional} wrapping a bounded {@link CdsAction}.
*
* @param name the name of the {@code CdsAction}
* @return an {@code Optional} describing the {@code CdsAction} with the given
* name, or an empty {@code Optional} if there is no {@code CdsAction}
* with this name
*/
Optional findAction(String name);
/**
* Returns a sequential {@code Stream} over the bounded {@link CdsFunction
* CdsFunction(s)} of this {@code CdsEntity}.
*
* @return a sequential {@code Stream} over the {@code CdsFunction(s)}
*/
Stream functions();
/**
* Get a {@link CdsFunction} via its name.
*
* @param name the name of the {@code CdsFunction}
* @return the {@code CdsFunction} with the given name
* @throws CdsOperationNotFoundException if there is no {@code CdsFunction} with
* the given name
*/
CdsFunction getFunction(String name);
/**
* Returns an {@link Optional} wrapping a bounded {@link CdsFunction}.
*
* @param name the name of the {@code CdsFunction}
* @return an {@code Optional} describing the {@code CdsFunction} with the given
* name, or an empty {@code Optional} if there is no {@code CdsFunction}
* with this name
*/
Optional findFunction(String name);
@Override
default CdsKind getKind() {
return CdsKind.ENTITY;
}
@Override
default void accept(CdsVisitor visitor) {
elements().forEach(e -> e.accept(visitor));
visitor.visit(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy