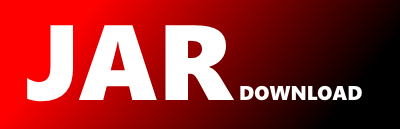
com.sap.cds.CdsData Maven / Gradle / Ivy
The newest version!
/************************************************************************
* © 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
************************************************************************/
package com.sap.cds;
import java.util.Map;
/**
* Represents data of CDS objects, which can be accessed as a
* {@code Map} and hold additional metadata.
*
* To simplify access to nested data representing deep structures, path access
* is supported via {@link #containsPath}, {@link #putPath}, {@link #getPath}
* and {@link #removePath}. CdsData can conveniently be serialized to JSON using
* {@link #toJson()}.
*/
public interface CdsData extends Map, JSONizable {
Factory factory = Cds4jServiceLoader.load(Factory.class);
/**
* Creates a new {@link CdsData} instance.
*
* @return an empty CdsData instance
*/
static CdsData create() {
return factory.create();
}
/**
* Returns a {@link CdsData} instance backed by the given map.
*
* @param backingMap the backing data map
* @return a {@link CdsData} instance backed by backingMap
*/
static CdsData create(Map backingMap) {
return factory.create(backingMap);
}
/**
* Returns the value to which the specified key is mapped. If no value is found,
* null is returned, see {@link Map#get(Object)}.
*
* @param key the key whose associated value is to be returned
* @return the value to which the specified key is mapped, or null if this map
* contains no mapping for the key
*/
@Override
Object get(Object key);
/**
* Returns the value to which the specified path (see {@link #containsPath}) is
* mapped. If no value is found, null is returned. Dots in the path are
* interpreted as separators, use {@link #get} to access values with keys that
* contain dots.
*
* @param path the path whose associated value is to be returned
* @param the type of the associated value
* @return the value to which the specified path is mapped, or null if this map
* contains no mapping for the path
*/
T getPath(String path);
/**
* Returns the value to which the specified path (see {@link #containsPath}) is
* mapped, or defaultValue if this map contains no mapping for the path. Dots in
* the path are interpreted as separators, use {@link Map#getOrDefault} to
* access values with keys that contain dots.
*
* @param path the path whose associated value is to be returned
* @param defaultValue the default mapping for the path
* @param the type of the associated value
* @return the value to which the specified path is mapped, or defaultValue if
* this map contains no mapping for the path
*/
T getPathOrDefault(String path, T defaultValue);
/**
* Associates the specified value with the specified path in this map. If the
* map previously contained a mapping for the path ({@link #containsPath} is
* true), the old value is replaced by the specified value. Creates nested maps
* along the path, if a path segment (except for the last segment) is not
* mapped.
*
* @param path the path with which the specified value is to be associated
* @param value the value to be associated with the specified path
* @param the type of the associated value
* @return the previous value associated with the path, or null if there was no
* mapping for the path
*/
T putPath(String path, T value);
/**
* If the specified path (see {@link #containsPath}) is not already associated
* with a value (or is mapped to null) associates it with the given value and
* returns null, else returns the current value. Creates nested maps along the
* path, if a path segment (except for the last segment) is not mapped.
*
* @param path the path with which the specified value is to be associated
* @param value the value to be associated with the specified path
* @param the type of the associated value
* @return the previous value associated with the path, or null if there was no
* mapping for the path
*/
T putPathIfAbsent(String path, T value);
/**
* Returns true if this map contains a mapping for the specified path. A path
* consists of one or many segments separated by a dot, every path segment is
* used as key of a nested map.
*
* @param path the path to a nested map's key whose presence is to be tested
* @return true if this or the nested map addressed by the path contains a
* mapping for the specified key
*/
boolean containsPath(String path);
/**
* Removes the mapping for a path (see {@link #containsPath}) from this map if
* it is present (optional operation). Returns the value previously associated
* with the path, or null if the map contained no mapping for the path.
*
* The map will not contain a mapping for the specified path once the call
* returns.
*
* @param path the path whose mapping is to be removed from the map
* @return the previous value associated with the path, or null if there was no
* mapping for the path.
*/
T removePath(String path);
/**
* Marks this data instance for removal inside of a
* {@link CdsList#delta() delta list}.
*
* @return this data instance
*/
default T forRemoval() {
return forRemoval(true);
}
/**
* Marks or unmarks this data instance for removal inside of a
* {@link CdsList#delta() delta list}.
*
* @param remove true to mark for removal, false to unmark
*
* @return this data instance
*/
T forRemoval(boolean remove);
/**
* Indicates if this data instance is marked for removal inside of a
* {@link CdsList#delta() delta list}.
*
* @return true if this instance if marked for removal
*/
boolean isForRemoval();
/**
* Returns the metadata value to which the specified key is mapped.
* If no value is found, null is returned.
* Metadata key-value pairs are not stored in the {@link #entrySet()} of this {@link Map}.
*
* @param key the key whose associated metadata value is to be returned
* @param the type of the associated metadata value
* @return the metadata value to which the specified key is mapped, or null if there was no
* mapping for the key
*/
T getMetadata(String key);
/**
* Associates the specified metadata value with the specified key.
* Metadata key-value pairs are not stored in the {@link #entrySet()} of this {@link Map}.
*
* @param key the key with which the specified metadata value is to be associated
* @param value the metadata value to be associated with the specified key
* @param the type of the associated metadata value
* @return the previous metadata value associated with the key, or null if there was no
* mapping for the key
*/
T putMetadata(String key, T value);
public interface Factory {
CdsData create();
CdsData create(Map other);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy