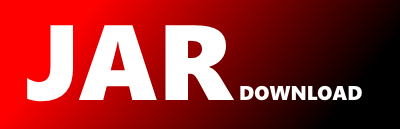
com.sap.cds.ql.cqn.CqnExpression Maven / Gradle / Ivy
The newest version!
/*******************************************************************
* © 2019 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.ql.cqn;
import java.util.stream.Collectors;
public interface CqnExpression extends CqnValue {
@Override
default boolean isExpression() {
return true;
}
@Override
default CqnExpression asExpression() {
return this;
}
/**
* Returns {@code true} if this is a {@link CqnArithmeticExpression}.
*
* @return {@code true} if this is an arithmetic expression, otherwise
* {@code false}
*/
default boolean isArithmeticExpression() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnCaseExpression}.
*
* @return {@code true} if this is a case expression, otherwise
* {@code false}
*/
default boolean isCaseExpression() {
return false;
}
/**
* Casts this expression to a {@code CqnArithmeticExpression}.
*
* @throws ClassCastException if this expression is not an arithmetic expression
* @return this as an arithmetic expression
*/
default CqnArithmeticExpression asArithmeticExpression() {
throw new ClassCastException("Cannot cast to CqnArithmeticExpression");
}
/**
* Casts this expression to a {@code CqnCaseExpression}.
*
* @throws ClassCastException if this expression is not a case expression
* @return this as a case expression
*/
default CqnCaseExpression asCaseExpression() {
throw new ClassCastException("Cannot cast to CqnArithmeticExpression");
}
/**
* Returns {@code true} if this is a {@link CqnArithmeticNegation}.
*
* @return {@code true} if this is a negation expression, otherwise
* {@code false}
*/
default boolean isNegation() {
return false;
}
/**
* Casts this expression to a {@code CqnArithmeticNegation}.
*
* @throws ClassCastException if this expression is not an arithmetic negation
* @return this as a negation expression
*/
default CqnArithmeticNegation asNegation() {
throw new ClassCastException("Cannot cast to CqnArithmeticNegation");
}
/**
* Returns {@code true} if this is a {@link CqnPredicate}.
*
* @return {@code true} if this is a predicate, otherwise {@code false}
*/
default boolean isPredicate() {
return false;
}
/**
* Casts this expression to a {@code CqnPredicate}.
*
* @throws ClassCastException if this expression is not a predicate
* @return this as a predicate
*/
default CqnPredicate asPredicate() {
throw new ClassCastException("Cannot cast to CqnPredicate");
}
@Override
default String toJson() {
return "[" + tokens().map(CqnToken::toJson).collect(Collectors.joining(", ")) + "]";
}
@Override
default void accept(CqnVisitor visitor) {
visitor.visit(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy