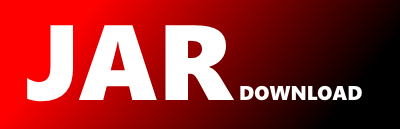
com.sap.cds.ql.cqn.CqnStatement Maven / Gradle / Ivy
/************************************************************************
* © 2019-2022 SAP SE or an SAP affiliate company. All rights reserved. *
************************************************************************/
package com.sap.cds.ql.cqn;
import java.util.Map;
import com.sap.cds.CdsException;
import com.sap.cds.JSONizable;
public interface CqnStatement extends JSONizable {
/**
* Returns the reference of this statement.
*
* {@link CqnSelect} statements do not necessarily have a reference, e.g. a
* select from subquery. This can be checked via {@link CqnSelect#from()}.
*
* @throws CdsException if this statement does not have a reference
* @return the reference
*/
CqnStructuredTypeRef ref();
/**
* Returns the runtime hints of this statement.
*
* @return the runtime hints
*/
Map hints();
/**
* Returns {@code true} if this is a {@link CqnSelect} statement.
*
* @return {@code true} if this is a select statement, otherwise {@code false}
*/
default boolean isSelect() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnInsert} statement.
*
* @return {@code true} if this is an insert statement, otherwise {@code false}
*/
default boolean isInsert() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnUpsert} statement.
*
* @return {@code true} if this is an upsert statement, otherwise {@code false}
*/
default boolean isUpsert() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnUpdate} statement.
*
* @return {@code true} if this is an update statement, otherwise {@code false}
*/
default boolean isUpdate() {
return false;
}
/**
* Returns {@code true} if this is a {@link CqnDelete} statement.
*
* @return {@code true} if this is a delete statement, otherwise {@code false}
*/
default boolean isDelete() {
return false;
}
/**
* Casts this CQN statement to {@code CqnSelect}.
*
* @throws ClassCastException if this statement is not a select
* @return this statement as a CqnSelect
*/
default CqnSelect asSelect() {
throw new ClassCastException("Cannot cast to CqnSelect");
}
/**
* Casts this CQN statement to {@code CqnInsert}.
*
* @throws ClassCastException if this statement is not an insert
* @return this statement as a CqnInsert
*/
default CqnInsert asInsert() {
throw new ClassCastException("Cannot cast to CqnInsert");
}
/**
* Casts this CQN statement to {@code CqnUpsert}.
*
* @throws ClassCastException if this statement is not an upsert
* @return this statement as a CqnUpsert
*/
default CqnUpsert asUpsert() {
throw new ClassCastException("Cannot cast to CqnUpsert");
}
/**
* Casts this CQN statement to {@code CqnUpdate}.
*
* @throws ClassCastException if this statement is not an update
* @return this statement as a CqnUpdate
*/
default CqnUpdate asUpdate() {
throw new ClassCastException("Cannot cast to CqnUpdate");
}
/**
* Casts this CQN statement to {@code CqnDelete}.
*
* @throws ClassCastException if this statement is not a delete
* @return this statement as a CqnDelete
*/
default CqnDelete asDelete() {
throw new ClassCastException("Cannot cast to CqnDelete");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy