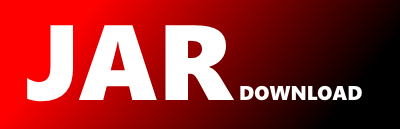
com.sap.cds.ql.cqn.ResolvedSegment Maven / Gradle / Ivy
/*******************************************************************
* © 2020 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.ql.cqn;
import java.util.Map;
import com.sap.cds.ql.cqn.CqnReference.Segment;
import com.sap.cds.reflect.CdsElement;
import com.sap.cds.reflect.CdsEntity;
import com.sap.cds.reflect.CdsStructuredType;
/**
* Contains information on a {@link Segment} of a {@link CqnStructuredTypeRef}
*/
public interface ResolvedSegment {
/**
* Returns the unmodified {@link Segment}.
*
* @return a Segment
*/
CqnReference.Segment segment();
/**
* Returns the {@link CdsEntity} that is referenced by the {@link Segment}.
*
* @return a {@code CdsEntity}
*/
default CdsEntity entity() {
return type().as(CdsEntity.class);
}
/**
* Returns the {@link CdsStructuredType} that is referenced by the
* {@link Segment}.
*
* @return the type referenced by the segment
*/
CdsStructuredType type();
/**
* Returns the {@link CdsElement} corresponding to this {@link Segment}.
*
* @return the {@code CdsElement}, or null
*/
CdsElement element();
/**
* Returns the element name to value mapping for the key elements of the
* {@link CdsEntity} that is targeted by the {@link Segment}.
*
* Extracts the key values, i.e. the values of the key elements to the entity
* targeted by this segment, from this segment's filter condition. If the filter
* condition does not uniquely restrict a key element to a particular value, the
* element's name is mapped to {@code null}.
*
* @return a map relating all key element names to key values
*/
Map keys();
/**
* Returns the element name to value mapping for the key elements of the
* {@link CdsEntity} that is targeted by the {@link Segment}.
*
* Extracts the key values, i.e. the values of the key elements to the entity
* targeted by this segment, from this segment's filter condition. If the filter
* condition does not uniquely restrict a key element to a particular value, the
* element's name is not present in the returned map.
*
* @return a map relating key element names to values
*/
Map keyValues();
/**
* Returns the element name to value mapping for all elements of the
* {@link CdsEntity} that is targeted by the {@link Segment}.
*
* Extracts the values, i.e. the values of the elements to the entity targeted
* by this segment, from this segment's filter condition. If the filter
* condition does not uniquely restrict an element to a particular value, the
* element's name is not present in the returned map.
*
* @return a map relating element names to values
*/
Map values();
}