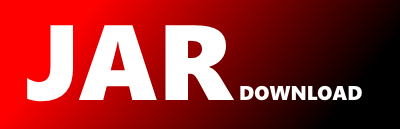
com.sap.cds.reflect.CdsAnnotatable Maven / Gradle / Ivy
The newest version!
/*******************************************************************
* © 2019 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.reflect;
import java.util.Optional;
import java.util.function.Predicate;
import java.util.stream.Stream;
import com.google.common.annotations.Beta;
public interface CdsAnnotatable {
/**
* Returns a sequential {@code Stream} over all {@link CdsAnnotation
* CdsAnnotation(s)} of this {@code CdsAnnotatable}.
*
* @return a sequential {@code Stream} over the {@code CdsAnnotation(s)}
*/
Stream> annotations();
/**
* Returns an {@link Optional} wrapping a {@link CdsAnnotation}.
*
* @param the expected type of the annotation value
* @param name the name of the {@code CdsAnnotation}
* @return an {@code Optional} describing the {@code CdsAnnotation} with the
* given name, or an empty {@code Optional} if there is no
* {@code CdsAnnotation} with this name
*/
Optional> findAnnotation(String name);
/**
* Returns the value of the {@code CdsAnnotation} with the given name.
*
* @param the type of the annotation value
* @param name the name of the {@code CdsAnnotation}
* @param defaultValue the value to be returned if there is no annotation
* present, may be null
* @return the value of the annotation, or {@code defaultValue} if there is no
* annotation with the given name
*/
@SuppressWarnings("unchecked")
default T getAnnotationValue(String name, T defaultValue) {
return (T) findAnnotation(name).map(CdsAnnotation::getValue).orElse(defaultValue);
}
/**
* Returns a {@link Predicate} to filter {@code CdsAnnotatable(s)} that have a
* {@link CdsAnnotation} with the given name.
*
* @param annotation the name of the {@code CdsAnnotation}
* @return a {@link Predicate} filtering by annotations
*/
static Predicate byAnnotation(String annotation) {
return a -> a.findAnnotation(annotation).isPresent();
}
@Beta
default Optional getDoc() {
return Optional.empty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy