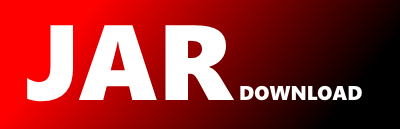
com.sap.cds.reflect.CdsParameter Maven / Gradle / Ivy
/*******************************************************************
* © 2019-2022 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.reflect;
import java.util.Optional;
public interface CdsParameter extends CdsAnnotatable {
/**
* Returns the name of this {@code CdsParameter}.
*
* @return the name of this parameter, not {@code null}
*/
String getName();
/**
* Returns the type of this {@code CdsParameter}.
*
* @return the type of this parameter, not {@code null}
*/
CdsType getType();
/**
* Returns an {@link Optional} wrapping the default value of this
* {@code CdsParameter}.
*
* @return an {@code Optional} describing the default value of the
* {@code CdsParameter}, or an empty {@code Optional} if there is no
* default value
*/
Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy