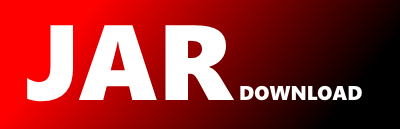
com.sap.cds.generator.util.FileSystem Maven / Gradle / Ivy
The newest version!
/************************************************************************
* © 2019-2024 SAP SE or an SAP affiliate company. All rights reserved. *
************************************************************************/
package com.sap.cds.generator.util;
import static java.nio.file.StandardCopyOption.REPLACE_EXISTING;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.nio.channels.Channels;
import java.nio.channels.ReadableByteChannel;
import java.nio.file.Files;
import java.nio.file.Path;
import org.apache.commons.io.IOUtils;
import org.slf4j.LoggerFactory;
public class FileSystem implements GeneratedFile.Accessor {
private final org.slf4j.Logger logger = LoggerFactory.getLogger(FileSystem.class);
private final Path baseDirectory;
private final boolean mavenLayout;
private final boolean overwrite;
public FileSystem(Path baseDirectory, boolean mavenLayout) {
this(baseDirectory, mavenLayout, true);
}
public FileSystem(Path baseDirectory, boolean mavenLayout, boolean overwrite) {
this.mavenLayout = mavenLayout;
this.overwrite = overwrite;
File dir = baseDirectory.toFile();
if (dir.exists() && !dir.isDirectory()) {
String message = "'%s' is not a directory.".formatted(baseDirectory.getFileName());
throw new IllegalArgumentException(message);
}
this.baseDirectory = baseDirectory;
}
@Override
public void accept(GeneratedFile file) throws IOException {
try {
Path outputPath = getPath(file);
if (Files.exists(outputPath)) {
if (!overwrite) {
logger.info("Class {} already exists. No new handler is generated.", outputPath.getFileName());
} else {
try (ReadableByteChannel rbc = Files.newByteChannel(outputPath);
InputStream in = Channels.newInputStream(rbc)) {
if (!IOUtils.contentEquals(file.getContent(), in)) {
Files.copy(file.getContent(), outputPath, REPLACE_EXISTING);
}
}
}
} else {
Files.createDirectories(outputPath.toFile().getParentFile().toPath());
Files.copy(file.getContent(), outputPath, REPLACE_EXISTING);
}
} catch (IOException e) {
String message = "Error writing file '%s' to file system.".formatted(file.getUri());
throw new IOException(message, e);
}
}
private Path getPath(FileLocation location) {
Path base = getBase(location);
return base.resolve(location.getUri().getPath());
}
private Path getBase(FileLocation location) {
if (!mavenLayout) {
return baseDirectory;
}
return baseDirectory.resolve(location.isResource() ? "resources" : "java");
}
@Override
public GeneratedFile get(FileLocation location) throws IOException {
Path path = getPath(location);
return new GeneratedFile() {
@Override
public URI getUri() {
return location.getUri();
}
@Override
public InputStream getContent() throws IOException {
if (!path.toFile().exists()) {
return null;
}
return Files.newInputStream(path);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy