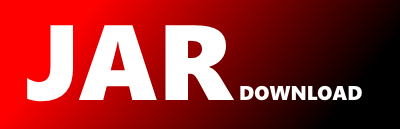
com.sap.cds.impl.localized.LocaleUtils Maven / Gradle / Ivy
/*******************************************************************
* © 2019 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.impl.localized;
import static com.sap.cds.reflect.impl.reader.model.CdsConstants.CDS_LOCALIZED;
import java.util.Locale;
import java.util.Optional;
import com.sap.cds.reflect.CdsAnnotation;
import com.sap.cds.reflect.CdsElement;
import com.sap.cds.reflect.CdsEntity;
/**
* Utility class to handle the locale settings
*/
public class LocaleUtils {
private LocaleUtils() {
}
/**
* Prefix for the localized entities and views
*/
private static final String LOCALIZED_PREFIX = "localized";
/**
* Calculates the localized entity name that can be used to access localized
* fields
*
* @param entity the entity
* @return the localized entity name
*/
public static String localizedEntityName(CdsEntity entity) {
return LOCALIZED_PREFIX + "." + entity.getQualifiedName();
}
/**
* Calculates the localized entity name that can be used to access localized
* fields
*
* @param entity the entity name
* @return the localized entity name
*/
public static String localizedEntityName(String entity) {
return LOCALIZED_PREFIX + "." + entity;
}
/**
* Calculates the localized entity name for the specific locale that can be used
* to access localized fields
*
* @param entity the entity name
* @param locale the locale
* @return the localized entity name
*/
public static String localizedEntityName(String entity, Locale locale) {
return LOCALIZED_PREFIX + "." + locale.getLanguage() + "." + entity;
}
/**
* Checks if a given entity name is a localized entity name
*
* @param entity the entity name
* @return true if the given name if a localized entity name
*/
public static boolean isLocalizedEntityName(String entity) {
return entity.startsWith(LOCALIZED_PREFIX + ".");
}
/**
* Calculates the locale String value from a specific locale that can be used to
* access localized attributes fields
*
* @param locale the locale
* @return the locale String value
*/
public static String getLocaleString(Locale locale) {
if (locale != null) {
String localeStr = locale.getLanguage();
String country = locale.getCountry();
if (!country.isEmpty()) {
localeStr += "_" + country;
}
String extension = locale.getExtension('x');
if (extension != null && !extension.isEmpty()) {
localeStr += "_" + extension;
}
return localeStr;
}
return null;
}
public static boolean isLocalized(CdsEntity entity) {
Optional> map = entity.findAnnotation(CDS_LOCALIZED);
if (map.isPresent()) {
if (!map.get().getValue()) {
return false;
}
}
return entity.concreteNonAssociationElements().anyMatch(CdsElement::isLocalized);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy