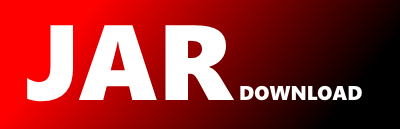
com.sap.cds.impl.parser.XsertParser Maven / Gradle / Ivy
/*******************************************************************
* © 2019 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.impl.parser;
import java.util.List;
import java.util.Map;
import com.jayway.jsonpath.DocumentContext;
import com.jayway.jsonpath.TypeRef;
import com.sap.cds.ql.Insert;
import com.sap.cds.ql.Upsert;
import com.sap.cds.ql.cqn.CqnStructuredTypeRef;
import com.sap.cds.ql.cqn.CqnSyntaxException;
import com.sap.cds.ql.cqn.CqnXsert;
import com.sap.cds.ql.impl.XsertBuilder;
public class XsertParser extends CqnParser {
private final CqnXsert.Kind kind;
private static final TypeRef>> LIST_OF_MAPS = new TypeRef>>() {
};
private XsertParser(CqnXsert.Kind kind, DocumentContext cqn) {
super(cqn);
this.kind = kind;
}
public static Insert parseInsert(String cqn) {
XsertParser parser = new XsertParser(CqnXsert.Kind.INSERT, getDocumentContext(cqn));
return XsertBuilder.insert(parser.ref()).entries(parser.entries());
}
public static Upsert parseUpsert(String cqn) {
XsertParser parser = new XsertParser(CqnXsert.Kind.UPSERT, getDocumentContext(cqn));
return XsertBuilder.upsert(parser.ref()).entries(parser.entries());
}
private CqnStructuredTypeRef ref() {
return TokenParser.ref(cqn.read("$." + kind + ".into"));
}
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy