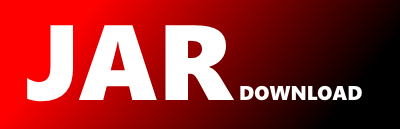
com.sap.cds.ql.impl.XsertBuilder Maven / Gradle / Ivy
/*******************************************************************
* © 2019 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.ql.impl;
import static com.sap.cds.impl.builder.model.StructuredTypeImpl.structuredType;
import static com.sap.cds.impl.builder.model.StructuredTypeRefImpl.typeRef;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
import java.util.stream.Stream;
import com.fasterxml.jackson.databind.node.ArrayNode;
import com.sap.cds.impl.parser.token.AbstractJSONizable;
import com.sap.cds.impl.parser.token.Jsonizer;
import com.sap.cds.ql.Insert;
import com.sap.cds.ql.StructuredType;
import com.sap.cds.ql.StructuredTypeRef;
import com.sap.cds.ql.Upsert;
import com.sap.cds.ql.cqn.CqnInsert;
import com.sap.cds.ql.cqn.CqnModifier;
import com.sap.cds.ql.cqn.CqnStructuredTypeRef;
import com.sap.cds.ql.cqn.CqnUpsert;
import com.sap.cds.ql.cqn.CqnVisitor;
import com.sap.cds.ql.cqn.CqnXsert;
import com.sap.cds.util.DataUtils;
public class XsertBuilder extends AbstractJSONizable {
private final CqnXsert.Kind kind;
private final StructuredType> entity;
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy