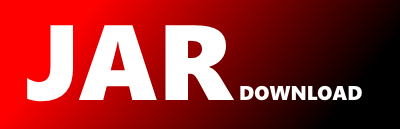
com.sap.cds.reflect.impl.CdsElementBuilder Maven / Gradle / Ivy
/*******************************************************************
* © 2020 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.reflect.impl;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.stream.Collectors;
import com.sap.cds.reflect.CdsAnnotation;
import com.sap.cds.reflect.CdsDefinition;
import com.sap.cds.reflect.CdsElement;
import com.sap.cds.reflect.CdsType;
public class CdsElementBuilder extends CdsAnnotableBuilder {
private String name;
private final CdsTypeBuilder typeBuilder;
public CdsTypeBuilder getTypeBuilder() {
return typeBuilder;
}
private T type;
private final boolean isKey;
private final boolean isVirtual;
private final boolean isNotNull;
private final boolean isLocalized;
private CdsDefinitionBuilder> declaringType;
public CdsElementBuilder(List> annotations, String name, CdsTypeBuilder typeBuilder,
boolean isKey, boolean isVirtual, boolean isNotNull, boolean isLocalized) {
super(annotations);
this.name = name;
this.typeBuilder = typeBuilder;
this.isKey = isKey;
this.isVirtual = isVirtual;
this.isNotNull = isNotNull;
this.isLocalized = isLocalized;
}
public static CdsElementBuilder element(String name) {
return new CdsElementBuilder<>(Collections.emptyList(), name, null, false, false, false, false);
}
public static CdsElementBuilder> copy(CdsElement element) {
List> annotations = element.annotations().collect(Collectors.toList());
return new CdsElementBuilder<>(annotations, element.getName(), null, element.isKey(), element.isVirtual(),
element.isNotNull(), element.isLocalized()).type(element.getType());
}
public CdsElementBuilder name(String name) {
this.name = name;
return this;
}
public CdsElementBuilder type(T type) {
this.type = type;
return this;
}
public String getName() {
return name;
}
void setDeclaringType(CdsDefinitionBuilder> declaringType) {
if (this.declaringType == null) {
this.declaringType = declaringType;
}
}
public CdsElement build(CdsDefinition declarator) {
if (type == null) {
type = typeBuilder.build();
}
return new CdsElementImpl(annotations, name, type, isKey, isVirtual, isNotNull, isLocalized, declarator);
}
class CdsElementImpl extends CdsAnnotatableImpl implements CdsElement {
private final String name;
private final T type;
private final boolean isKey;
private final boolean isVirtual;
private final boolean isNotNull;
private final boolean isLocalized;
private final CdsDefinition declarator;
public CdsElementImpl(Collection> annotations, String name, T type, boolean isKey,
boolean isVirtual, boolean isNotNull, boolean isLocalized, CdsDefinition declarator) {
super(annotations);
this.name = name;
this.type = type;
this.isKey = isKey;
this.isVirtual = isVirtual;
this.isNotNull = isNotNull;
this.isLocalized = isLocalized;
this.declarator = declarator;
}
@Override
public String getName() {
return name;
}
@Override
@SuppressWarnings("unchecked")
public D getDeclaringType() {
return (D) declarator;
}
@Override
@SuppressWarnings("unchecked")
public T getType() {
return type;
}
@Override
public boolean isKey() {
return isKey;
}
@Override
public boolean isVirtual() {
return isVirtual;
}
@Override
public boolean isUnique() {
throw new UnsupportedOperationException();
}
@Override
public boolean isNotNull() {
return isNotNull;
}
@Override
public boolean isLocalized() {
return isLocalized;
}
@Override
public String toString() {
return name;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy