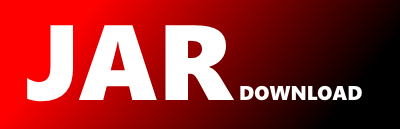
com.sap.cds.reflect.impl.CdsEventBuilder Maven / Gradle / Ivy
/*******************************************************************
* © 2020 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.reflect.impl;
import static com.sap.cds.reflect.impl.CdsStructuredTypeBuilder.noSuchElement;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.stream.Stream;
import com.sap.cds.reflect.CdsAnnotation;
import com.sap.cds.reflect.CdsElement;
import com.sap.cds.reflect.CdsEvent;
public class CdsEventBuilder extends CdsDefinitionBuilder {
private final List> elements = new ArrayList<>();
public CdsEventBuilder(List> annotations, String qualifiedName) {
super(annotations, qualifiedName);
}
void addElement(CdsElementBuilder> e) {
this.elements.add(e);
}
void addElements(List> elements) {
elements.forEach(this::addElement);
}
@Override
public CdsEvent build() {
return new CdsEventImpl(annotations, qualifiedName, elements);
}
private static class CdsEventImpl extends CdsDefinitionImpl implements CdsEvent {
private final Map elements = new HashMap<>();
private CdsEventImpl(Collection> annotations, String qualifiedName,
List> ebs) {
super(annotations, qualifiedName);
ebs.stream().filter(e -> !e.toBeIgnored(this.getQualifiedName() + "." + e.getName()))
.forEach(eb -> this.elements.put(eb.getName(), eb.build(this)));
}
@Override
public Stream elements() {
return elements.values().stream();
}
@Override
public Optional findElement(String name) {
return Optional.ofNullable(elements.get(name));
}
@Override
public CdsElement getElement(String name) {
return findElement(name).orElseThrow(noSuchElement("element", name, this.getQualifiedName()));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy