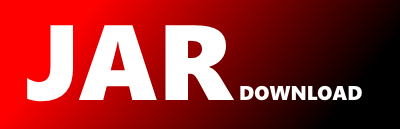
com.sap.cds.reflect.impl.CdsParameterBuilder Maven / Gradle / Ivy
/*******************************************************************
* © 2020 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.reflect.impl;
import java.util.Collection;
import java.util.List;
import java.util.Optional;
import java.util.function.Function;
import com.sap.cds.reflect.CdsAnnotation;
import com.sap.cds.reflect.CdsParameter;
import com.sap.cds.reflect.CdsType;
public class CdsParameterBuilder extends CdsAnnotableBuilder {
private final String name;
private final CdsTypeBuilder> type;
private final Function defaultValueProvider;
public CdsParameterBuilder(List> annotations, String name, CdsTypeBuilder> type, Function defaultValueProvider) {
super(annotations);
this.name = name;
this.type = type;
this.defaultValueProvider = defaultValueProvider;
}
public CdsParameter build() {
CdsType cdsType = type.build();
return new CdsParameterImpl(annotations, getName(), cdsType, defaultValueProvider.apply(cdsType));
}
/**
* @return the name
*/
public String getName() {
return name;
}
private static class CdsParameterImpl extends CdsAnnotatableImpl implements CdsParameter {
private final String name;
private final CdsType type;
private final Object defaultValue;
private CdsParameterImpl(Collection> annotations, String name, CdsType type, Object defaultValue) {
super(annotations);
this.name = name;
this.type = type;
this.defaultValue = defaultValue;
}
@Override
public String getName() {
return name;
}
@Override
public CdsType getType() {
return type;
}
@Override
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy