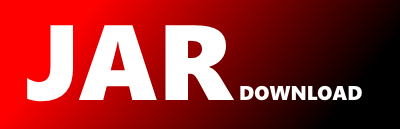
com.sap.cds.reflect.impl.CdsUnboundActionAndFunctionReader Maven / Gradle / Ivy
/*********************************************************************
* (C) 2020 SAP SE or an SAP affiliate company. All rights reserved. *
*********************************************************************/
package com.sap.cds.reflect.impl;
import static com.sap.cds.reflect.impl.CdsModelReader.findType;
import static com.sap.cds.reflect.impl.CdsModelReader.readType;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.function.Function;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.fasterxml.jackson.databind.JsonNode;
import com.sap.cds.CdsException;
import com.sap.cds.impl.parser.TokenParser;
import com.sap.cds.reflect.CdsType;
import com.sap.cds.reflect.impl.reader.issuecollector.IssueCollector;
import com.sap.cds.reflect.impl.reader.issuecollector.IssueCollectorFactory;
import com.sap.cds.reflect.impl.reader.model.CdsConstants;
public class CdsUnboundActionAndFunctionReader {
private static final Logger logger = LoggerFactory.getLogger(CdsUnboundActionAndFunctionReader.class);
private static final IssueCollector issueCollector = IssueCollectorFactory
.getIssueCollector(CdsUnboundActionAndFunctionReader.class);
private CdsUnboundActionAndFunctionReader() {
}
public static CdsActionBuilder readAction(String qualifiedName, JsonNode csn) {
return new CdsActionBuilder(CdsAnnotationReader.read(csn), qualifiedName);
}
public static CdsFunctionBuilder readFunction(String qualifiedName, JsonNode csn) {
return new CdsFunctionBuilder(CdsAnnotationReader.read(csn), qualifiedName);
}
// used in CdsModelReader
public static List readParameterList(String name, JsonNode jsonStructuredType,
CdsModelBuilder model) {
List paramList = new LinkedList<>();
JsonNode params;
if (jsonStructuredType.has(CdsConstants.PARAMS)) {
params = jsonStructuredType.get(CdsConstants.PARAMS);
} else {
return paramList;
}
Iterator> fields = params.fields();
while (fields.hasNext()) {
Entry next = fields.next();
JsonNode field = next.getValue();
String paramName = next.getKey();
try {
CdsTypeBuilder> type = findType(field, model)
.orElseGet(() -> readType("", field, model));
Function defValProvider = (t) -> TokenParser.defaultValue(field.get(CdsConstants.DEFAULT), t);
paramList.add(new CdsParameterBuilder(CdsAnnotationReader.read(field), paramName, type, defValProvider));
} catch (InvalidCsnException e) {
String pathToElement = name + "." + paramName;
logger.debug(pathToElement + ": ", e);
issueCollector.error(pathToElement, e.getMessage());
}
}
return paramList;
}
public static CdsTypeBuilder> readReturnType(JsonNode jsonStructuredType, CdsModelBuilder model) {
if (jsonStructuredType.has(CdsConstants.RETURNS)) {
JsonNode returns = jsonStructuredType.get(CdsConstants.RETURNS);
return findType(returns, model).orElseGet(() -> readType("", returns, model));
} else if (jsonStructuredType.get(CdsConstants.KIND).asText().equalsIgnoreCase(CdsConstants.FUNCTION)) {
throw new CdsException("Missing return type function. Please add the return type in the CDS model.");
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy