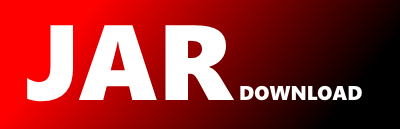
com.sap.cds.impl.parser.StructDataParser Maven / Gradle / Ivy
/*******************************************************************
* © 2020 SAP SE or an SAP affiliate company. All rights reserved. *
*******************************************************************/
package com.sap.cds.impl.parser;
import static com.sap.cds.impl.parser.CqnParser.parseJson;
import static java.util.stream.Collectors.toList;
import static java.util.stream.StreamSupport.stream;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.node.ArrayNode;
import com.sap.cds.ql.CdsDataException;
import com.sap.cds.reflect.CdsArrayedType;
import com.sap.cds.reflect.CdsAssociationType;
import com.sap.cds.reflect.CdsEntity;
import com.sap.cds.reflect.CdsSimpleType;
import com.sap.cds.reflect.CdsStructuredType;
import com.sap.cds.reflect.CdsType;
import com.sap.cds.util.CdsTypeUtils;
public class StructDataParser {
private final CdsStructuredType rootType;
private StructDataParser(CdsStructuredType type) {
this.rootType = type;
}
public static StructDataParser create(CdsStructuredType type) {
return new StructDataParser(type);
}
public static List> parseArrayOf(CdsType itemType, String jsonArray) {
if (itemType.isSimple()) {
return parseArrayOf(itemType.as(CdsSimpleType.class), jsonArray);
}
if (itemType.isStructured()) {
return parseArrayOf(itemType.as(CdsStructuredType.class), jsonArray);
}
throw new IllegalArgumentException("Cannot parser array of type " + itemType);
}
@SuppressWarnings("unchecked")
public static List parseArrayOf(CdsSimpleType itemType, String jsonArray) {
if (jsonArray != null) {
ArrayNode data = parseJson(jsonArray);
return (List) arrayToList(data, itemType);
}
return null;
}
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy